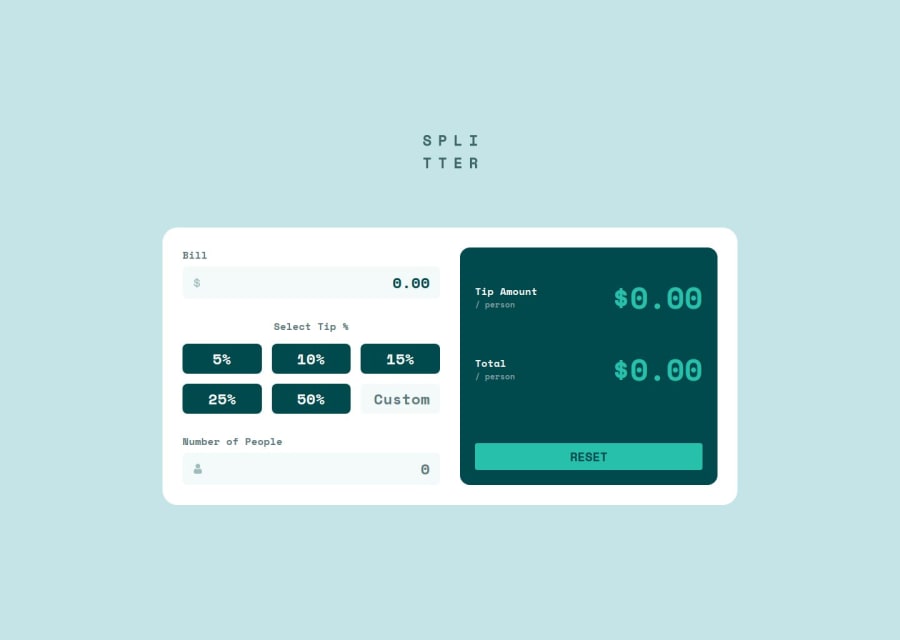
Tip Calculator App using modular javascript and BEM
Design comparison
Solution retrospective
- Debouncing implementation that significantly improves calculator performance:
export const debounce = (func, wait) => {
let timeout;
return function executedFunction(...args) {
const later = () => {
clearTimeout(timeout);
func(...args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
};
This elegant solution prevents excessive calculations during rapid user input, making the calculator more efficient.
- Robust number formatting system that handles all edge cases:
export const formatters = {
currency: (input) => {
// Formatting Logic
},
percentage: (input) => {
// Formatting Logic
}
};
- Clean modular code structure with separate concerns (validation, utils, events)
-
Start with BEM methodology from the beginning: Instead of refactoring later, implementing BEM from the start would create a more maintainable structure and avoid the need to rename classes and restructure HTML/CSS later in development. This would save time and ensure consistent component organization throughout the project.
-
Implement keyboard navigation accessibility features earlier: Building accessibility from the ground up would create a more inclusive application and prevent the need to retrofit keyboard controls. This includes proper tab ordering, ARIA labels, and keyboard shortcuts for all interactive elements.
-
Create more reusable components from the start: Breaking down the calculator into smaller, reusable components initially would make the code more modular and easier to maintain. The number formatting and validation systems could be designed as standalone modules that could be used in other projects.
These changes would improve the development workflow and result in a more robust final product.
What specific areas of your project would you like help with?- Is the current debounce timing (150ms) optimal for user experience?
- How could the BEM structure be improved further for better component isolation?
- Could the validation system be more robust while maintaining its simplicity?
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord