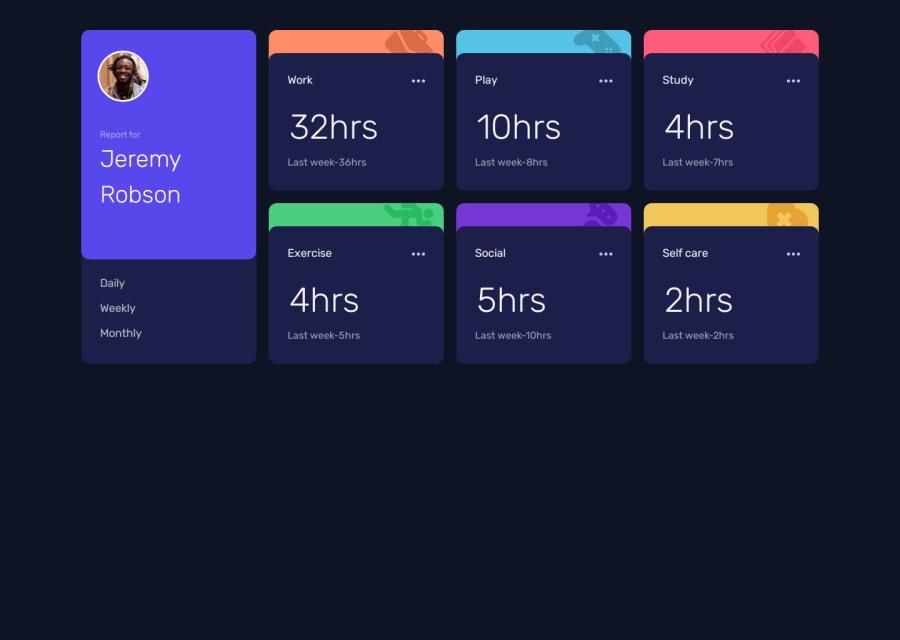
Design comparison
Community feedback
- @skyv26Posted 2 months ago
Hi @Hlm19966,
You've done a great job so far! The foundation of your code is solid, but I have a few suggestions that could improve readability, maintainability, and user experience. Let's refine it together! 🚀
1. Refactor JavaScript Code
- Make your code functional and reusable to reduce duplication and enhance modularity.
- Use
async/await
instead of.then
for better readability and modern JavaScript practices. - Write compact and clean code to make it easier for others to understand.
Suggested Refactored Code:
let globalData = []; const fetchData = async () => { try { const response = await fetch('./data.json'); if (!response.ok) throw new Error('Error fetching JSON'); globalData = await response.json(); console.log(globalData); } catch (error) { console.error('Error:', error); } }; const updateDisplay = (data, timeframes) => { const hours = document.querySelectorAll('.hours'); const previousHours = document.querySelectorAll('.previous-hours'); data.forEach((item, index) => { hours[index].innerHTML = `${item.timeframes[timeframes].current}hrs`; previousHours[index].innerHTML = `Last: ${item.timeframes[timeframes].previous}hrs`; }); }; ['daily', 'weekly', 'monthly'].forEach((period) => { document.getElementById(period).addEventListener('click', () => updateDisplay(globalData, period)); }); // Fetch the data on page load fetchData();
Improvements:
- Reusability: The
updateDisplay
function can handle all timeframes dynamically. - Readability:
async/await
simplifies the promise handling. - Error Handling: Clear and consistent error messages.
- Performance: Removed redundant event listener code.
2. Use Arrow Functions and Shorthand Syntax
Replace traditional functions with arrow functions to improve compactness and adhere to modern JavaScript practices. 🌟
Example:
const logData = () => console.log(globalData);
3. Center Dashboard Vertically
Your
body
styling is almost perfect for centering the dashboard. Just tweak the flex direction for complete alignment:body { margin: 0; padding: 0; box-sizing: border-box; overflow-x: hidden; display: flex; flex-direction: column; justify-content: center; align-items: center; min-height: 100vh; }
This will ensure a balanced vertical and horizontal center alignment for the dashboard. 🎨
These changes will make your code more modern, concise, and easier for others to understand and maintain. Feel free to share your updated code so we can review and refine it further. 😊
Keep up the great work! 💪
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord