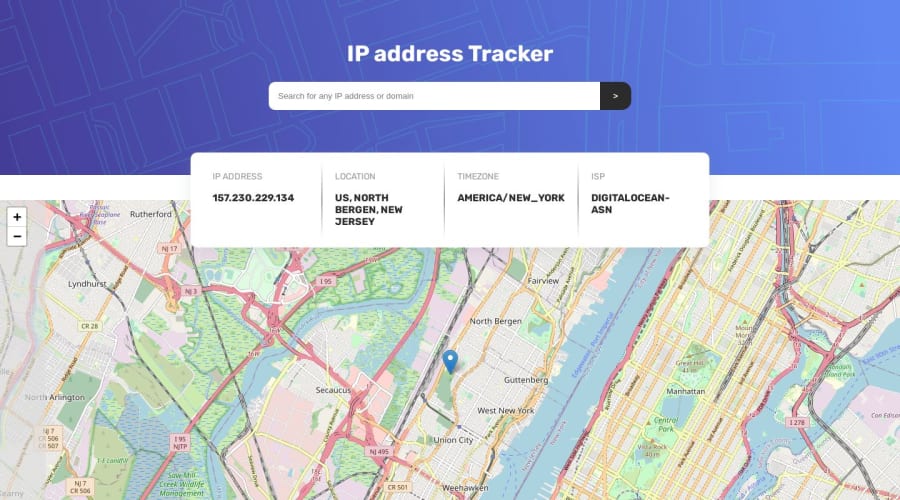
Design comparison
Solution retrospective
It was good practice to strengthen my React skills, although I encountered a problem with the search button click. With React I didn't know how to implement the button click and read the value from the input field, I just implemented it to listen for a keyPress "Enter".
Maybe someone could guide me how to implement and solve this issue. :)
Community feedback
- @Dev-MV6Posted over 1 year ago
You can use the
onClick
event handler on the search button. You just need to refactor theSearchBar
component and use a single function to handle both events.Here's a refactored version of your
SearchBar
component:import { useRef } from "react"; function SearchBar({ setSearchValue }) { const searchBoxRef = useRef(null); function onSearchValue() { const searchBox = searchBoxRef.current; setSearchValue(searchBox.value); // Clear input box after searching searchBox.value = ""; } return ( <div> <input ref={searchBoxRef} type="text" placeholder="Search for any IP address or domain" onKeyDown={e => e.key === "Enter" ? onSearchValue() : null} ></input> <span> <button className="search-btn" onClick={onSearchValue}>></button> </span> </div> ); } export default SearchBar;
Don't forget to pass the
setSearchValue
callback to the component:<SearchBar setSearchValue={ setSearchValue } />
You can read more about
onClick
anduseRef
in the React documentation:Hope you find this helpful 👍
Marked as helpful0@An-RenataPosted over 1 year ago@Dev-MV6 Hi! It helped! I appreciate that you took the time to open my source code and provide a solution to my problem. I'm truly grateful.
I still always wonder where this useRef() hook is useful. Step by step I will finally master it. :D
Thanks again!
0@Dev-MV6Posted over 1 year ago@An-Renata I'm glad to help 👋
From the React documentation: "
useRef
is a React Hook that lets you reference a value that’s not needed for rendering."When you declare a variable inside a component React will update it every time it renders the component, so what can you do if you need to store some value that doesn't change on every render (like a DOM element)?
useRef
hook solves this issue:You can call
useRef
hook and pass the value you want the ref object’s current property to be initially. It can be a value of any type or evennull
:const intervalRef = useRef(0);
And whenever you want to access the value stored inside the ref object you use the
current
property:console.log(intervalRef.current);
You can use the
useRef
hook to store a DOM element by setting the reference object as the value of theref
attribute of the element you want to store:import { useRef } from "react"; function SearchBox() { const inputRef = useRef(null); return <input ref={inputRef} />; }
Now if you have a function that needs to access the value of the
input
element:function getInputValue() { // the current property holds the element const input = inputRef.current; // now you can access the element normally const value = input.value; console.log(value); }
I hope I've made myself clear. Let me know if you have any further questions.
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord