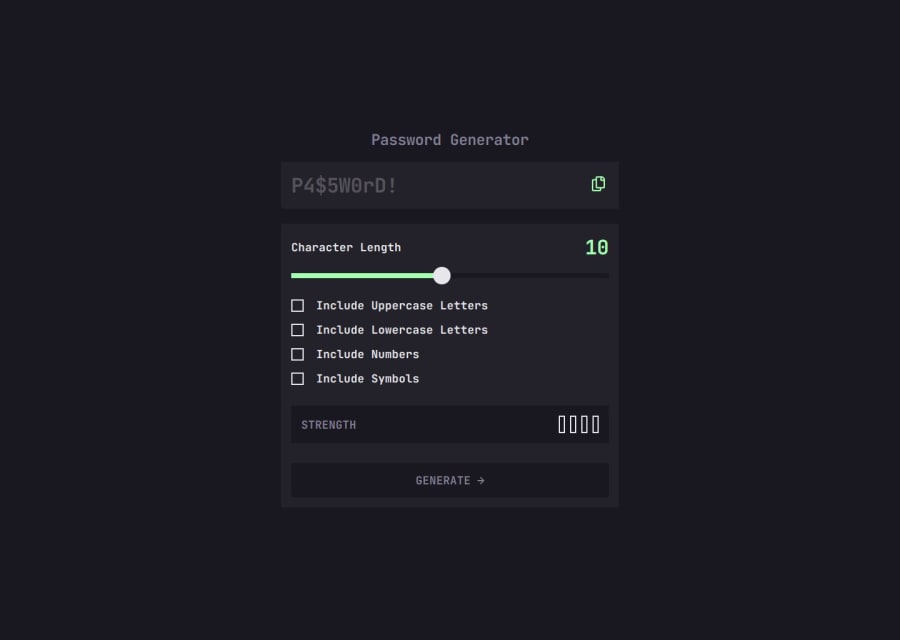
Password Generator App with custom notification funcionality
Design comparison
Solution retrospective
I'm most proud of how I implemented the dynamic password strength calculation system.
For example, this part of the code made me proud:
export const calculateStrength = () => {
setStrength(strengths.filter((input) => input.checked).length);
if (lengthValue < 12) return strength - 1;
if (lengthValue > 16 && strength > 0) return strength + 1;
return strength;
};
This implementation dynamically calculates password strength based on both the number of selected character types and password length, providing immediate visual feedback through the strength indicator bars.
What challenges did you encounter, and how did you overcome them?The real-time slider animation with dynamic color gradients was a challenging aspect to implement correctly. I solved this by creating a function that calculates the percentage and updates the background gradient:
export const runSliderAnimation = () => {
const value =
((lengthSlider.value - lengthSlider.min) /
(lengthSlider.max - lengthSlider.min)) *
100;
lengthSlider.style.background = `linear-gradient(to right, var(--clr-neon-green) ${value}%, var(--clr-very-dark-gray) ${value}%)`;
};
What specific areas of your project would you like help with?
I would love feedback on the following areas:
Password Generation Algorithm:
The current implementation is basic and could be enhanced. How can I make it more secure and ensure better distribution of character types? Here's the current generation function:
export const generatePassword = () => {
const charset = [
"ABCDEFGHIJKLMNOPQRSTUVWXYZ",
"abcdefghijklmnopqrstuvwxyz",
"0123456789",
"!@#$%^&*()_+-=[]{}|;:,.<>?/"
];
const selectedCharsets = strengths
.map((input, index) => input.checked ? charset[index] : '')
.filter(set => set !== '')
.join('');
let password = "";
for (let i = 0; i < lengthValue; i++) {
const randomIndex = Math.floor(Math.random() * selectedCharsets.length);
password += selectedCharsets.charAt(randomIndex);
}
return password;
};
Should I implement a more sophisticated algorithm that ensures at least one character from each selected type is included?
Accessibility Implementation:
While I've included ARIA attributes and screen reader support, I'm particularly interested in feedback on the strength indicator implementation:
<div class="strength" role="region" aria-label="Password strength indicator"> <p class="strength__label">Strength</p> <div class="strength__meter"> <p class="strength__value" id="strengthValue" aria-live="polite"></p> <div class="strength__indicator" id="strengthIndicator" role="progressbar" aria-label="Password strength bars"> <div class="strength__bar"></div> <div class="strength__bar"></div> <div class="strength__bar"></div> <div class="strength__bar"></div> </div> </div> </div>
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord