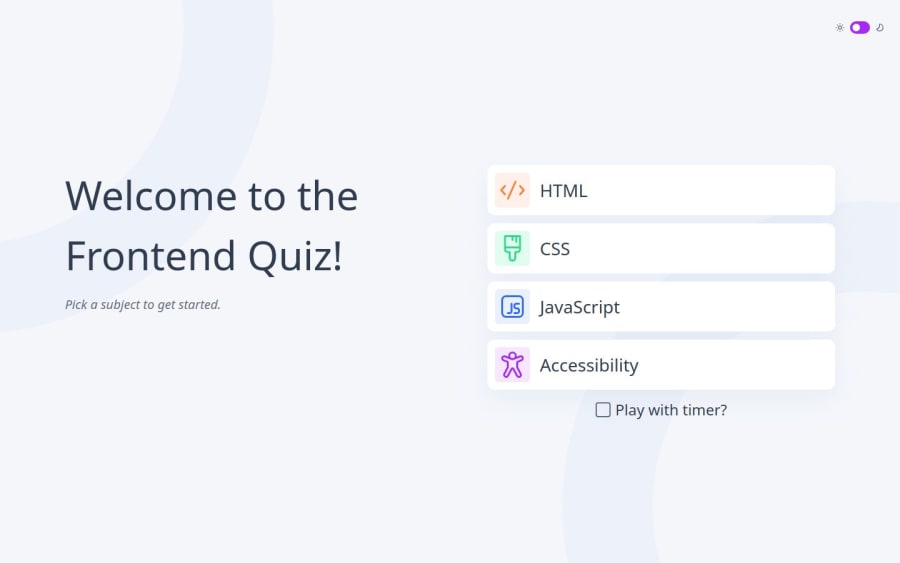
Design comparison
Solution retrospective
I'm most proud of how I implemented the timer functionality in the quiz application. For example, this part of the code made me proud:
export const setTimer = () => {
const duration = getTimerDuration();
if (!duration || !elements.timerCheck.checked) {
elements.root.style.setProperty("--animate-timer-playstate", "paused");
saveTimerSettings();
return;
};
state.timerDuration = duration;
elements.root.style.setProperty("--animate-timer-playstate", "running");
elements.root.style.setProperty("--animate-timer-duration", `${state.timerDuration}s`);
saveTimerSettings();
};
This implementation uses CSS variables and animations for the timer visualization rather than JavaScript intervals, which is more performant and smoother. I also implemented persistent timer and theme settings using localStorage, allowing users to maintain their preferences between sessions.
What challenges did you encounter, and how did you overcome them?One of the most significant challenges I encountered was implementing the quiz selection and rendering system that could handle different quiz categories dynamically. The main difficulty was creating a structure that could load the appropriate quiz data based on user selection and render it correctly. I solved this by developing a modular approach using the quiz-data.js and quiz-renderer.js files:
import {createQuiz} from "./quiz-renderer.js";
export const quizTypes = {
html: (quiz) => createQuiz(quiz),
css: (quiz) => createQuiz(quiz),
javascript: (quiz) => createQuiz(quiz),
accessibility: (quiz) => createQuiz(quiz),
};
This approach allowed me to maintain a clean separation of concerns between data fetching, quiz creation, and rendering logic. The quiz selection handler in quiz-logic.js then ties everything together:
export const handleQuizSelection = (event) => {
const button = event.target.closest(".menu__button");
if (!button) return;
state.currentQuiz = button.textContent.trim();
updateHeader();
const selectedQuiz = quizTypes[state.currentQuiz.toLowerCase()];
const quiz = selectedQuiz
? selectedQuiz(getQuizData(state.currentQuiz))
: null;
quiz ? renderQuiz(quiz) : restoreMainMenu();
};
Another challenge was implementing the dark/light theme toggle that needed to work seamlessly with the rest of the UI. The theme switching functionality required careful consideration of accessibility and user preferences, ensuring that all UI elements remained visible and properly styled in both themes.
What specific areas of your project would you like help with?I would love feedback on the following areas:
- Timer Implementation in Quiz App
The timer functionality in my quiz app has been challenging to implement correctly. Here's the relevant code from my timer.js file:
import {elements, quizElements} from "./elements.js";
export const setTimesUpEvent = () => {
// This function is called when the quiz is rendered
// I need help ensuring this properly handles the timer animation
// and correctly triggers when time runs out
}
I'm particularly concerned about:
-
How to properly sync the CSS animation with the JavaScript timer logic
-
Ensuring the timer resets correctly between questions
-
Handling edge cases when a user answers just as time runs out
- Quiz Selection and Rendering Logic
I'm also looking for feedback on my quiz selection and rendering approach:
export const handleQuizSelection = (event) => {
const button = event.target.closest(".menu__button");
if (!button) return;
state.currentQuiz = button.textContent.trim();
updateHeader();
const selectedQuiz = quizTypes[state.currentQuiz.toLowerCase()];
const quiz = selectedQuiz
? selectedQuiz(getQuizData(state.currentQuiz))
: null;
quiz ? renderQuiz(quiz) : restoreMainMenu();
};
Is this approach maintainable for adding new quiz types in the future? Should I be using a different pattern for handling the quiz selection and creation?
Community feedback
- P@nishanth1596Posted 7 days ago
Hi @Crtykwod,
Awesome work on this project! The timer feature is a great addition. 🎉
I just noticed that the timer runs for the whole quiz instead of resetting for each question, which makes it a bit tricky to complete the quiz properly. I’m not too experienced with JS, but I think the timer might need to reset when moving to the next question. Maybe you could check that?
Also, for better project organization, creating a separate repo for each project would be ideal. Branching is usually used for feature updates rather than separate projects.
One more thing—since this wasn’t done in React, it might be better not to tag React for clarity.
And if possible, adding a bit more margin to the theme switcher would make the UI even better!
Great job overall! Looking forward to seeing more of your work. 🚀
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord