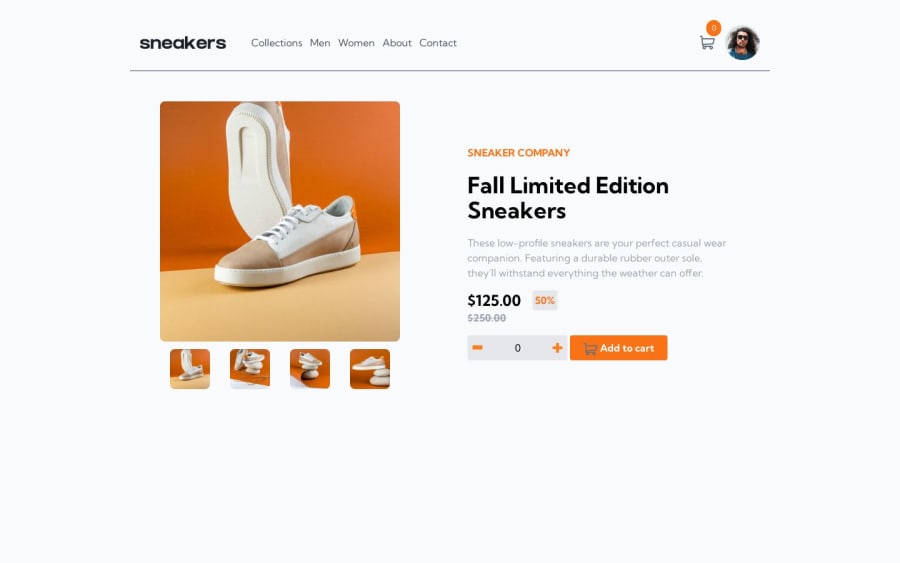
Design comparison
Solution retrospective
These are the two main issues that I'm facing. Will be glad to get a pointer as to what I'm doing wrong: Updating the total cost price- I get a nan on the total cost price of the product Deleting a product from the cart - the remove button doesn't seem to be triggered. Was working fine before
Community feedback
- @florianstancioiuPosted almost 2 years ago
Hi Mubaraq,
Here are some tips to make your code more readable.
-
First off, install prettier for vscode (I'm assuming you use Visual Studio Code, if not, please install prettier for your text editor of choice). https://marketplace.visualstudio.com/items?itemName=esbenp.prettier-vscode
-
Secondly, try to separate the JavaScript logic in different files (You can create a
slider.js
for the slider and acart.js
file for the cart), writting all JS code inside one giant file is a big mistake and should be avoided. -
Thirdly, writting HTML strings directly to DOM is prone to security exploits like XSS. You can aleviate this by using
<template></template>
tags or by using a template engine. Here are some resources on using the template tag: https://javascript.info/template-element , https://www.w3schools.com/tags/tag_template.asp
(Don't lose too much sleep over the third tip)
Now let's address your issues:
- Updating the total cost price- I get a nan on the total cost price of the product
The reason why you get
NaN
is because the string starts with a$
, and that turns intoNaN
. The simplest solution is to remove the$
character usingproductPrice.substring(1)
.// If the product is not in the cart, add it as a new cart item const cartItem = { // ... totalPrice: Number(productPrice.substring(1)) * Number(productCount), };
- Deleting a product from the cart - the remove button doesn't seem to be triggered. Was working fine before
The reason why it didn't work is because the click event is targeting the image element, and the image element doesnt have the
.remove-button
class, so we have to look for the nearest/closest element with that class.To fix it, replace this line:
if (event.target.classList.contains('remove-button')) { // ... }
with
if (event.target.closest('.remove-button')) { // ... // And comment this function call bellow, otherwise you will get an error: // updateCartTotalPrice() }
As a last note, don't create functions inside event handlers, create the functions in the global scope. Also, don't create event listeners inside event listeners.
// BAD addToCartButton.addEventListener('click', () => { function updateCartItemCount() {} function updateCartItem(cartItem) {} function updateCartTotalPrice() {} cartIcon.addEventListener('click', () => {}); });
// GOOD addToCartButton.addEventListener('click', () => {}); function updateCartItemCount() {} function updateCartItem(cartItem) {} function updateCartTotalPrice() {} cartIcon.addEventListener('click', () => {});
I think you will still face some issues after tweaking the code using my advice, especially with the cart. Please don't take my advice the wrong way, I'm only trying to help you out. I hope this will help you a bit in your journey, keep on coding!
Florian
Marked as helpful2@muubaraqPosted almost 2 years agoThank you very much. Never in my wildest dream will take and advice in a wrong way. How then will I be able to learn and improve. Will separate the files and hopefully sort it out. Been on it for like 3 days now. Wanted to really do it by myself before asking for help. 😂😂@florianstancioiu
2 -
- @ssenyondo67Posted almost 2 years ago
Hi, I have enjoyed viewing your implementation but when I was interacting with the cart I found out the following. The Item is added to the cart but the total price is NaN this is because you converted the product price containing "$" a string that can't be converted to a number, you can use regular expressions(.replace(/$/g,'') to remove it. And when you submit the product again the cart item number changes but the cart item itself does not change also the delete functionality in the cart is not working because of the way you access the item by using the "dataset.title" yet you set "data-index". Anyway, nice work done so far looking forward to seeing your updates.
Marked as helpful1@muubaraqPosted almost 2 years agoThank you for this feedback. Will definitely look into it@ssenyondo67
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord