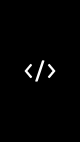
Shahin Aliyarli
@sliyarliAll comments
- @aviralsharma07Submitted about 1 year ago@sliyarliPosted about 1 year ago
Sure, here are some suggestions to help improve the code provided:
-
HTML Structure and Semantics:
- Make sure to close all HTML tags properly. For example, some of your input tags are missing the closing angle bracket.
- Consider using semantic HTML tags like
<header>
,<nav>
,<section>
,<article>
, and<footer>
for better document structure and accessibility.
-
CSS Styling:
- Use CSS variables for colors, fonts, and other repeated values to make it easier to update the styling throughout your application.
- Organize your CSS styles into meaningful classes and IDs to improve readability and maintainability.
- Consider using a CSS preprocessor like Sass or Less to simplify your stylesheets and make them more modular.
-
Responsive Design:
- Ensure your webpage is responsive by using media queries. Test it on various screen sizes and devices to ensure it looks good and functions correctly.
-
JavaScript:
- Separate your JavaScript code into functions for better code organization and readability.
- Consider using event delegation to handle form validation more efficiently.
- For email validation, you can use the HTML5
type="email"
attribute on the email input field to ensure a valid email format.
-
Error Handling:
- Improve the error handling mechanism. Right now, it displays errors below each input field. You could consider using a single error message area that dynamically updates with relevant error messages.
-
Comments and Documentation:
- Add comments to your code to explain its functionality, especially in JavaScript. It will make it easier for you or others to understand the code in the future.
-
Form Validation:
- Currently, you are checking for errors when the user clicks the "Claim your free trial" button. Consider adding real-time validation as the user types to provide immediate feedback.
-
Accessibility:
- Ensure your website is accessible to users with disabilities. Use appropriate ARIA attributes and labels for form elements.
-
Testing:
- Thoroughly test your form on different browsers and devices to ensure cross-browser compatibility.
-
Performance:
- Optimize your images and assets for faster loading times.
- Consider using a Content Delivery Network (CDN) for common libraries like Google Fonts to improve page load speed.
-
Version Control:
- Consider using version control systems like Git to track changes in your codebase and collaborate more effectively.
-
Security:
- Implement server-side validation and security measures to protect against malicious input.
-
User Experience (UX):
- Enhance the user experience by providing clear feedback when the form is submitted successfully.
Remember that coding is an ongoing learning process, and there's always room for improvement. These suggestions should help you enhance your code and skills.
0 -
- @masha-a-mSubmitted about 1 year ago
DAY 1-5 20 DAYS OF CODE CHALLENGE HerTechTrail
Day 1-5 Details
This is a repo for Day 1-5 of 20 days of code challenge HerTechTrail. For Day 1-5 we were required to replicate a given sign up form, style it with CSS, make it responsive and also host it.
Table of content
Project Task
Users should be able to:
- sign up with the form
Links
- Solution URL (https://github.com/masha-a-m/day1-5_20_Days_of_Code_Challenge_HerTechTrail)
- Live Site URL (https://lucky-biscuit-5c0c3a.netlify.app)
My Process
I started this project a week ago. It was a bit hectic for me but with research on different sites, I finally finished it.
Built With
- HTML5 Markup
- CSS Styling
- JavaScript
What I Learnt
I leant how to make a sign up form with many CSS components
Useful Resources
https://codereview.stackexchange.com/questions/114760/student-registration-form
https://stackoverflow.com/questions/20260798/creating-registration-form
Author
- Website - MARYAM GARBA
- Twitter - MARYAM GARBA
@sliyarliPosted about 1 year agoYour HTML and CSS code looks good for the most part, but I noticed a couple of issues and some room for improvements:
HTML Issues:
1 - In your HTML form, there are missing closing angle brackets (
>
) for the input elements - For example:<input type="text" placeholder="First Name" id="first"
Should be:
<input type="text" placeholder="First Name" id="first">
2 - The "Terms and Services" text within the
<span>
in your HTML should be properly closed with</span>
- Update this part:<p> By clicking the button, you are agreeing to our <span> Terms and Services</span> </p>
To:
<p> By clicking the button, you are agreeing to our <span> Terms and Services</span>. </p>
CSS Issues:
1 - There's a typo in the CSS for the
box-sizing
property - It should bebox-sizing
, but you haveboz-sizing
:*{ padding:0; margin:0; boz-sizing: border-box; /* should be box-sizing */ font-family: 'Poppins', sans-serif; }
JavaScript Issues:
1 - There's a typo in your JavaScript code when checking the
firstName
variable - You have:var firstname = first.value.trim();
It should be consistent with your variable name:
var firstName = first.value.trim();
2 - In the JavaScript code, you have this block of code duplicated for first name validation:
if (firstName === ''){ errorFunc(first, 'First Name cannot be empty') } else { successFunc(first) }
You should replace the second
successFunc(first)
withsuccessFunc(last)
for the last name validation.3 - In the
successFunc
function, you should also remove thesuccess
class when an input is in error state - You can do this by usingclassList.remove('success')
in addition to adding theerror
class.With these adjustments, your code should work correctly - Make sure to test it thoroughly to ensure everything is functioning as expected.
Marked as helpful0 - @Deep-910Submitted about 1 year ago@sliyarliPosted about 1 year ago
Here's a review of your code to help you improve your coding skills:
HTML:
- You have a well-structured HTML document with appropriate use of semantic tags.
- You've included meta tags for character set and viewport settings, which is good for responsive design.
- The usage of external CSS and JavaScript files is a good practice for code organization and maintainability.
CSS:
- You've used custom properties (CSS variables) effectively for defining colors and font sizes. This allows for easy customization.
- Your CSS follows a clear naming convention and is organized logically.
- Media queries have been used to make the layout responsive, which is great for ensuring your site looks good on different devices.
JavaScript:
- You've implemented input validation using JavaScript, which is an essential feature for forms.
- Your event listener for input validation is well-structured and efficient.
- The use of
getElementsByClassName
to select elements is correct, but you could also consider usingquerySelector
for more flexibility. - You've provided meaningful class names and IDs for your elements, making it easier to work with them in JavaScript.
Overall, your code demonstrates good coding practices. To further enhance your coding skills:
- Consider adding comments to your JavaScript code to explain the purpose of functions and code blocks.
- You could optimize your CSS for better performance by combining styles for similar elements.
- Explore more advanced JavaScript techniques and libraries to add interactivity and enhance user experience on your projects.
- Test your code thoroughly, especially when dealing with forms and user input, to ensure it behaves as expected.
Keep up the good work, and continue practicing and learning to become an even better developer!
Marked as helpful1 - @f1zziSubmitted about 1 year ago@sliyarliPosted about 1 year ago
Certainly, let's focus on suggestions that will help improve your coding skills:
1 - Code Organization and Readability: You've done a good job organizing your code into HTML, CSS, and JavaScript sections. Keep this practice consistent in larger projects to make your code more readable and maintainable.
2 - CSS Styling: Your CSS styling is clear and well-structured. Continue to use meaningful class names and comments to describe the purpose of each style rule. This will make it easier to understand and modify your styles in the future.
3 - Font Import: Importing Google Fonts as you did is a good practice for using custom fonts in your projects. Make sure to include comments explaining the purpose of imported fonts, especially when using multiple fonts.
4 - JavaScript Validation: Your JavaScript code for form validation is a good starting point. To improve your coding skills, consider expanding this validation to check for a valid email format and strong password criteria. This will give you valuable experience in handling user input.
5 - Code Comments: While your current code doesn't have many comments, adding comments is an excellent practice for improving your coding skills. In larger projects or when collaborating with others, comments help explain your code's logic and functionality.
6 - Responsive Design: Learning to create responsive designs is an essential skill for front-end developers. Continue practicing media queries to make your web pages adapt to different screen sizes and orientations. Experiment with different layouts and designs for various devices.
7 - Accessibility: Understanding and implementing accessibility principles in your code is crucial. Practice providing appropriate labels for form elements, ensuring keyboard navigation, and testing your pages with screen readers. This will enhance your skills in creating inclusive web experiences.
8 - Validation Feedback: Enhance your validation feedback. Instead of only changing the border color, consider displaying an error message next to the input field to provide users with clear feedback on what needs to be corrected.
9 - Testing: Always thoroughly test your code on various browsers and devices to ensure compatibility. This practice is vital for delivering a consistent user experience.
10 - Project Scope: Consider expanding the functionality of your project. For example, you could make the form functional by adding a server-side script to process submissions. This will give you valuable experience in handling user data.
By focusing on these suggestions, you'll not only improve your coding skills but also enhance the quality and functionality of your web projects. Keep practicing and exploring new techniques to continue growing as a front-end developer.
Marked as helpful0 - @ClaudioAmarenoSubmitted about 1 year ago
Hello, that's my solution of challenge called "stats-preview". Could you tell me what can I improve into my code? Thank you for any advice!
@sliyarliPosted about 1 year agoSure, let's provide feedback on @ClaudioAmareno's code:
1 - HTML Structure: The HTML structure is well-organized and semantic, using appropriate tags for the content.
2 - CSS Styling: Your CSS is well-structured and commented, making it easy to understand. Good job on using CSS variables for colors and font families; this enhances maintainability.
3 - Responsiveness: You have implemented responsive design using media queries, which is great. It ensures your site adapts well to different screen sizes. However, I noticed a minor issue in your CSS: in the
.card__img
class, you have a background color set. While this doesn't affect the layout, it might be unintentional.4 - Centering: You mentioned concerns about centering elements. Overall, your elements are well-centered, especially the text within the card. However, when the screen width increases beyond 992px (desktop view), the text alignment shifts to the left. This might be intentional, but if you want to center-align it in this view as well, you can add
text-align: center;
to the.card__text
class within the appropriate media query.5 - Images: You're using two images for different screen sizes, which is a good practice for performance. Just make sure you optimize the images for the web to reduce load times.
6 - Accessibility: Consider adding alternative text (alt tags) to your images for better accessibility. This helps screen readers and users with disabilities understand the content.
7 - Spacing: The spacing and padding within your card are consistent and visually appealing. Good use of white space.
8 - Font Size: Font sizes are well-adjusted for different screen sizes through media queries, ensuring readability.
9 - Typography: Your choice of fonts (Inter and Lexend Deca) complements the design and is legible.
10 - Colors: The color scheme is aesthetically pleasing and maintains good contrast for readability. The use of HSL values is a good approach for maintaining consistent colors while adjusting shades.
Overall, you've done an excellent job in creating a responsive and visually appealing card component. Keep up the good work! If you have any further questions or need additional assistance, feel free to ask.
0 - @SeNaTu24Submitted about 1 year ago
I found media query difficult while building. I am unsure of the responsiveness and the centering of elements.
@sliyarliPosted about 1 year ago1 - Media Queries: You've successfully implemented media queries to make your website responsive, which is great. The mobile responsiveness part is well-handled in your CSS.
2 - Centering Elements: To address your concern about centering elements, there's an opportunity for improvement. In your mobile responsiveness section, you've centered the text content within the card, which is good. However, you can enhance the alignment of the statistics section. Currently, it's stacked vertically, and you could center it horizontally by adding
text-align: center;
to the.card .stats
class. Additionally, you might consider adding some spacing between the individual statistics for better visual separation on mobile devices.3 - Aspect Ratio on Image: Regarding maintaining the aspect ratio of the image during the switch from mobile to desktop, it looks like you've used a background image with a fixed height on the
.img_bg
class. This approach might not ensure the image's aspect ratio is maintained. Instead, you could use an<img>
element and setwidth: 100%; height: auto;
to ensure the image adapts to different screen sizes while maintaining its aspect ratio. You can wrap this in a<div>
to control the background color overlay.Here's an example of how to modify your CSS for maintaining image aspect ratio:
.img_bg { width: 50%; background-color: rgba(170, 92, 219, 0.7); display: flex; align-items: center; } .img_bg img { width: 100%; height: auto; }
These changes should help you maintain aspect ratios and improve centering on mobile devices. Keep up the good work, and don't hesitate to reach out if you have more questions or need further assistance!
Marked as helpful0 - @kcredd-ySubmitted about 1 year ago
Making the website responsive was a big hurdle, I ended up writing two sets of CSS code using media query for mobile and desktop. But still for a tiny bit of resolution when it switches from mobile to desktop the image doesn't maintain the aspect ratio, please point out my mistake and let me know how should use the breakpoints instead of writing a whole set of CSS codes twice
@sliyarliPosted about 1 year agoYou've done a great job in creating a responsive design for your Stats preview card component. I understand your concern about maintaining the aspect ratio of the image when switching between mobile and desktop views. Here's how you can achieve that without writing two sets of CSS rules:
1 - Use a Single CSS File: Instead of maintaining two separate sets of CSS rules for mobile and desktop, you can use a single CSS file with media queries to target specific screen sizes. This will make your code more maintainable.
2 - Aspect Ratio for Images: To maintain the aspect ratio of the image, you can use the
aspect-ratio
property. This property is especially useful for responsive design. Here's how you can apply it to your image:img { aspect-ratio: 16 / 9; /* Set the aspect ratio to 16:9, adjust as needed */ max-width: 100%; border-radius: 0 0.5rem 0.5rem 0; }
By setting the aspect ratio to 16:9, the image will maintain this aspect ratio regardless of the screen size.
3 - Simplify Media Queries: Instead of specifying
min-width
andmax-width
in separate media queries, you can use a combination ofmin-width
andmax-width
within the same query to target a specific range. For example:@media screen and (min-width: 601px) and (max-width: 927px) { /* Styles for tablets and smaller desktops */ }
This way, you can create more granular breakpoints without duplicating code.
By applying these changes, you can maintain the aspect ratio of the image while simplifying your CSS and making it easier to manage in the long run. Your code looks clean and well-structured, and with these adjustments, it will become even more efficient. Great job!
Marked as helpful0 - @Supber909Submitted about 1 year ago@sliyarliPosted about 1 year ago
You've created a nice-looking 3-column layout with HTML and CSS. Overall, it looks good, but I have a few suggestions to enhance your code:
1 - Semantic HTML: You've used appropriate HTML elements like headings (
<h1>
), paragraphs (<p>
), and buttons (<button>
) which is great. However, consider using semantic HTML elements like<section>
for each column to improve the structure and make it more accessible.2 - Accessibility: Ensure that your website is accessible. For instance, you can add
alt
attributes to your images for screen readers.3 - Consistency in CSS: While your CSS is organized, try to keep a consistent order in your CSS properties (e.g., always put
background-color
beforepadding
), which makes it easier to read and maintain.4 - Responsive Design: Currently, your layout has a fixed width. Consider making it responsive by using relative units like percentages or using CSS media queries to adapt the layout for different screen sizes. This will make your site look good on various devices.
5 - Spacing and Typography: The spacing and typography look good. However, make sure to keep consistent spacing between elements and maintain a good balance of whitespace.
6 - Button Styling: The buttons look fine, but consider adding some hover effects to make them more interactive. For example, you can change the background color or add a subtle shadow when the user hovers over them.
Here's a revised version of your HTML and CSS with some of these suggestions implemented:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="style.css"> <title>Challenge</title> </head> <body> <div class="general"> <section class="box"> <img src="images/icon-sedans.svg" alt="Sedans"> <h1 class="sed">SEDANS</h1> <p class="ch">Choose a sedan for its affordability and excellent fuel economy. Ideal for cruising in the city or on your next road trip.</p> <button class="learn-more sedans">Learn More</button> </section> <section class="box"> <img src="images/icon-suvs.svg" alt="SUVs"> <h1 class="suv">SUVS</h1> <p class="ta">Take an SUV for its spacious interior, power, and versatility. Perfect for your next family vacation and off-road adventures.</p> <button class="learn-more suvs">Learn More</button> </section> <section class="box"> <img src="images/icon-luxury.svg" alt="Luxury"> <h1 class="lux">LUXURY</h1> <p class="cr">Cruise in the best car brands without the bloated prices. Enjoy the enhanced comfort of a luxury rental and arrive in style.</p> <button class="learn-more luxury">Learn More</button> </section> </div> </body> </html>
* { padding: 0; margin: 0; box-sizing: border-box; } body { background-color: white; display: flex; justify-content: center; align-items: center; height: 90vh; } .general { display: grid; grid-template-columns: repeat(3, 1fr); max-width: 1200px; width: 100%; border-radius: 15px; gap: 20px; padding: 20px; margin: 0 auto; } .box { background-color: #E28524; border-radius: 10px; padding: 20px; display: flex; flex-direction: column; align-items: center; text-align: center; } h1 { color: rgba(255, 255, 255, 0.899); margin-top: 20px; font-size: 24px; } p { color: rgba(255, 255, 255, 0.685); margin-top: 10px; margin-bottom: 20px; line-height: 1.5; font-size: 16px; } button.learn-more { color: white; background-color: inherit; border: none; padding: 15px 30px; border-radius: 25px; cursor: pointer; transition: background-color 0.3s ease; } button.learn-more:hover { background-color: rgba(255, 255, 255, 0.2); }
These changes aim to improve the structure, accessibility, and responsiveness of your code while maintaining the visual style you've created.
Marked as helpful0 - @sheezylionSubmitted about 1 year ago
one of the things i find difficult in this project is the media query, although i created the desktop version before the mobile version.
@sliyarliPosted about 1 year agoYour HTML and CSS code looks good overall, but there are a few suggestions and improvements you can make:
HTML:
1 - It's a good practice to provide a value for the
description
meta tag within the<head>
section for better SEO. For example:<meta name="description" content="Your description here">
.2 - In your anchor tags for "Learn More," consider adding
href="#"
to make them clickable links. This enhances user experience and accessibility.CSS:
1 - It's a good practice to organize your CSS properties alphabetically or in a structured manner to improve readability and maintainability.
2 - You can combine your media queries to avoid duplication and make your CSS cleaner. For example:
@media screen and (max-width: 480px) { .container { /* Your styles here for small screens */ } .container-1 { /* Your styles here for small screens */ } .container-3 { /* Your styles here for small screens */ } }
3 - Consider using more meaningful class names. Instead of
container-1
,container-2
, andcontainer-3
, you could use class names that reflect the content they represent, likesedans
,suvs
, andluxury
.4 - You can simplify your CSS by using a single class for the "Learn More" buttons and applying different colors based on the container. For example:
.info-button { font-family: 'Lexend Deca', sans-serif; background-color: white; margin: 50px 30px; width: 130px; height: 40px; line-height: 40px; text-align: center; border-radius: 20px; } .sedans .info-button { color: #E28524; } .suvs .info-button { color: #006971; } .luxury .info-button { color: #00403E; }
This way, you can apply the same styling to all buttons and only change the text color based on the container.
Overall, your code is well-structured and responsive. These suggestions should help you make it even better and more maintainable. Keep up the good work!
Marked as helpful0 - @Tolux001Submitted about 1 year ago
♈Hello, Coding Community
This is my solution for the Stats Preview Card
Had a lots of fun building this challenge under record time!
I also got to know about Google page speed Insight, which helped me in page speed optimization. I Scored 97% on Google Page speed Insights! 🤯🤭😬
Layout was built responsive via MOBILE FIRST WORKFLOW
Feel free to leave any feedback and help me to improve my solution (or) make the code clean!
I made some custom tweaks to my code` CUSTOM TWEAKS..🌐 : I used Global declaration for variables :root{} Added smooth box shadow that roll out the shadow every 4s.
Also some hovering on the card while in desktop mode. 👨💻 Follow me in my journey to finish all newbie challenges to explore solutions with custom features and tweaks Ill be happy to hear any feedback and advice!
@sliyarliPosted about 1 year agoYou've done a great job with your HTML and CSS for the 3-column preview card component! Your code is clean and well-structured. Here are some specific tips to further improve it:
1 - Use Semantic HTML: Your use of HTML elements is good, but consider using more semantic HTML5 elements like
<header>
,<nav>
,<section>
, and<footer>
to provide better structure to your page. For instance, you can wrap your header and footer content in these elements.2 - Add a Favicon Description: In your
<head>
section, you have a<meta name="description">
tag, but it's missing the description text. Add a description to improve SEO and accessibility.3 - Responsive Design: Your design is already responsive, which is great! Continue testing it on various devices and screen sizes to ensure it looks good everywhere.
4 - Font Loading: You're using custom fonts, which is good for design. However, consider using the
font-display: swap;
property in your@font-face
declarations. This ensures that if the custom fonts take some time to load, the browser will use fallback fonts in the meantime, preventing a "flash of unstyled text."5 - Consolidate CSS: While your CSS is organized, some properties are repeated across different sections. Consider consolidating common styles into a single class and applying those classes to the appropriate elements. This reduces redundancy and makes your code easier to maintain.
6 - Button Styling: You're using buttons with different background colors and hover effects. Consider creating a CSS class for button styles and applying it to all buttons. This makes it easier to maintain and change the button styles consistently.
7 - Accessibility: Ensure that your images have appropriate
alt
attributes. They should describe the image content for users who rely on screen readers.Overall, your code is in good shape, and these tips should help you refine it further. Keep up the excellent work!
1 - @Wael-OrrabySubmitted about 1 year ago
If you have any suggestions for code improvements, feel free to share! Your feedback is greatly appreciated!
Thanks! 😊
@sliyarliPosted about 1 year agoYour HTML and CSS code for the 3-column preview card component looks quite good, but there are some improvements you can make to enhance it further. Let's go through them:
HTML Structure:
- Consider adding a
<!DOCTYPE html>
declaration at the very beginning of your HTML document. It's good practice and ensures your document is parsed correctly.
CSS Improvements:
- Use meaningful class names: Your class names like
.blok
could be more descriptive. Consider using names that reflect the content better, like.card
or.card-container
. - Consolidate common styles: You have repeated styles for the anchor (
a
) tag in each block. You can create a common class for these styles and apply it to all three anchor tags to make your CSS more efficient. - Avoid using
min-height
for responsive design: Instead of settingmin-height
on your.blok
elements, you can control their height and spacing better by using padding and margins. - Keep media queries organized: Your media query for larger screens is somewhat scattered throughout your CSS. It's good practice to group your media queries together at the end of your stylesheet for better readability.
- Consider adding a hover effect for your icons: Adding a subtle hover effect on the icons (like changing opacity or adding a slight scale effect) can make your cards more interactive and engaging.
Accessibility:
- Add
alt
attributes to images: It's essential to provide descriptivealt
attributes for your images to improve accessibility. This helps users with disabilities understand the content of the images.
Responsive Design:
- Your design looks good on larger screens, but you might want to test it on smaller screens to ensure it remains responsive and user-friendly. You can use media queries to adjust styles for different screen sizes as you've already done for larger screens.
Here's an example of how you could improve your code:
<!-- Consider adding a DOCTYPE declaration --> <!DOCTYPE html> <html lang="en"> <head> <!-- meta tags and links --> </head> <body> <div class="card-container"> <div class="card"> <img src="./images/icon-sedans.svg" alt="icon-sedans" /> <h2>Sedans</h2> <p> Choose a sedan for its affordability and excellent fuel economy. Ideal for cruising in the city or on your next road trip. </p> <a class="learn-more" href="#">Learn More</a> </div> <!-- Repeat similar structure for SUVs and Luxury --> </div> <!-- attribution and closing body/html tags --> </body> </html>
/* CSS Reset and :root definitions */ body { /* Padding and background styles */ } .card-container { /* Flex and spacing for smaller screens */ } .card { /* Card styles */ } .card h2 { /* Heading styles */ } .card p { /* Paragraph styles */ } .learn-more { /* Common styles for Learn More buttons */ } /* Media query for larger screens */ @media (min-width: 1440px) { /* Styles for larger screens */ }
These are just suggestions to improve your code further. Overall, your project looks great, and these improvements will make it even better. Good job!
Marked as helpful0 - Consider adding a
- @Islandstone89Submitted about 1 year ago@sliyarliPosted about 1 year ago
Hey @Islandstone89,
I checked out your Frontend Mentor challenge project for the Order Summary Card, and I must say, you've done an excellent job! Your project looks polished and well-structured. Here are some specific tips to help you take your project to the next level:
1 - Font Preloading: Great job on using Google Fonts for typography! To optimize font loading and prevent delays, consider adding the
preload
tag for the font in the<head>
of your HTML. This ensures the font is ready when needed.2 - CSS Custom Properties: I love how you've used CSS custom properties (variables) for colors and font weights. This approach makes it easy to maintain a consistent design throughout your project. Keep up the good work!
3 - Responsive Design: Your use of media queries and the
viewport
meta tag shows that you've prioritized responsiveness. To ensure a flawless experience on all devices, test your project across various screen sizes, and make any necessary adjustments.4 - Hover Transitions: Your link hover transitions provide a nice touch of interactivity. To add a bit more flair, consider adding a smooth transition effect to the "Cancel Order" button similar to what you've done with the "Change" link.
5 - Accessibility: It's awesome to see your attention to accessibility! To enhance it even further, ensure that all images have descriptive
alt
text and that your HTML structure maintains semantic elements for improved screen reader compatibility.6 - CSS Flexbox Mastery: Your use of Flexbox for layout is spot-on. As you continue developing, experiment with Flexbox's
justify-content
andalign-items
properties to fine-tune your layouts.7 - Button Styling: Your button styles are clear and concise. To make the user experience more engaging, consider adding a subtle animation or transition effect when users interact with buttons, enhancing their visual feedback.
8 - Code Comments: While your code is clean, consider adding comments to sections that might benefit from additional explanation. This will be especially helpful for collaborators or for revisiting the project in the future.
9 - Optimized Images: Your use of images adds a nice visual touch. To further optimize your project's loading times, consider compressing your images using online tools or software like ImageMagick.
10 - Maintain Consistency: Keeping a consistent code style—such as aligning attributes and properties—enhances readability. Also, consider using consistent class naming conventions for a cohesive codebase.
Keep up the fantastic work! Your attention to detail and commitment to creating a polished project really shine through. I'm looking forward to seeing more of your projects in the future. Feel free to reach out if you have any questions or if you'd like more feedback.
Marked as helpful1