I found myself using multiple for loops to access similar data. Is there any way I can refactor this using less code?
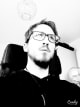
Rafal
@grizhlieCodesAll comments
- @ervinCodesSubmitted over 2 years ago@grizhlieCodesPosted over 2 years ago
Hi Ervin,
I made a video covering how I did this challenge, a lot of people seem to have found it useful. I'm pretty sure I explain the JS as I'm going through it.
In case you want a sneak peak of the code, here is the link to my GitHub repo.
Generally there is nothing wrong with using a for loop though.
The alternative you can try is the forEach loop.
It works like a normal for loop in a way, it usually takes in an array of items (lets say cards or buttons) and you can just run any code you want.
You have access to the index of each item as well as the item itself so for example:
buttons.forEach((button, index) => { button.textContent = `Button num ${index + 1}`; })
Whether or not it uses less code: probably. But I'd concern myself with readability personally rather than length. The less work your future self/someone else has to do to understand the code, the better. I've seen people obsess over quantity of code and they sometimes go as far as to sacrificing readability (I'm not saying you do this!), and man it's tough to work out what's what. Especially in the evening after a bunch of other work 🥲
Happy learning 👍!
P.S. I go into some CSS that you may find useful in the video for some additional tips.
1 - @bague-rodnelSubmitted almost 3 years ago
I'm not good with color theory. For now my thought process. is "just switch to light/dark shade of the same color and try to match how 'far' the color is from black/white". lol.
Share some good resources about the topic. :)
@grizhlieCodesPosted almost 3 years agoSuper awesome for you to practice extra stuff with this project, that's the spirit I LOVE (i tend to maximise my learning per project also).
When it comes to color, I struggle also.
From what I can tell is that you can't simply 'reverse' colors and hope for the best. Sort of like the complete invert-standard that seems to be prevelant amongst newer designers/developers. You need to find a similar 'theme' color and build around it. It can also be said that for this project you might have kept this card identical, even though the background changed since it's a nice contrast.
Imagine you had 3 cards, this would be the center, and the cards on the left/right would be the ones with the white/light-gray background. All of a sudden you've made this stand out massively. I'm just saying it's not as simple as one may think.
Feel free to criticise my website's dark/light mode toggle and tell me what you think. I think we can discuss and learn from the criticism. I'll be honest, I spent 5% of my time implementing the light mode and coloring so it probably sucks. I just wanted it to seem bearable.
I also like discussing stuff :).
An interesting resource is material ui (I think this is basically a google built team that creates AMAZING resources and guidelines for us devs/designers). They cover their thinking/principles/fundementals in incredible and useful detail :)
Marked as helpful1 - @RiscloverSubmitted almost 3 years ago
As always, feedback is much appreciated!
This took a little longer for everything to come together, but near the end, I got on a roll where I was able to figure out nearly all of the more difficult aspects pretty quickly (the images in desktop mode, the arrows turning upside down when you click on a question, etc). I'm glad I got to practice some JavaScript!
As a side note, I'm using a TV as an external monitor because my laptop screen is broken. Unfortunately, that means that sometimes my projects can look smaller to you guys than it does to me. My proportions are correct on my end, but I'm afraid that everything translates to be a bit small for everyone else. I have to figure that out. -_-
@grizhlieCodesPosted almost 3 years agoOverall a great solution, well done on everything! I agree with Rod on all points - hope you get yourself a new tool/fix your screen too :)
I think it would be more optimal to centre the images (Desktop view) in the vertical centre. If you open up all of the suggestions it leaves this odd white-space on the bottom of the images that I have to throw evil-eyes at 🤩.
If you want to practice even more javascript here, animate the anwsers to the questions when you click on a question. It's a fun exercise and I learned a ton from it that I honestly thought I already knew hehe.
To me transitions/animations are pretty important, almost as a part of accessibility now so I usually pay special attention to them. I also just find it 'nice'. If you need any advice/help with that just let me know. Or look at my solution for this to get a better idea. I was very.. let's call it specific with naming my JS variables just so I could later understand my code. It felt basically written in English by the end of it lol.
0 - @grizhlieCodesSubmitted almost 3 years ago
Hey all,
(Question on the bottom, summary on the top).
Just one I did for fun. I realised I could practice building keyboard-accessible navigation with some thought given to tabindex and when to add/remove it from the collapsible links. Then I realised how badly I wrote my JS and refactored it to be more readable.
I gave special attention to the transitions/animations for the header. You will see the collapsible menu-categories transition themselves from the direction/location of the buttons that activate them. I also added smooth transitions for the actual link-containers as well, the
max-height
,gap
,padding
andmargin
properties together is what makes the smoothness possible.Also learned about what exactly scrollHeight is and that it does not seem to include some aspects of the content, I believe it was omitting/excluding
line-height
of my<a>
's for example. It also ignores things like thegap
property of any parent flex container. So I just wrote my owncustomScrollHeight
variable. All in all I didn't expect to learn so much, as minor as it may seem at first. AWESOME project.I paid attention to making sure the grids I used were smooth. I did NOT pay attention to the background image for the hero, just sort of threw it in there with rough calculations, I'm sorry :(.
Question: Does anyone have any good thinking-framework for working with CSS grid? Some sort of a mental model with which to follow and break grid-down? My current style is based on analysing the design and then just fitting the grid in, mobile first with an OK understanding of the various grid-units we can use for rows/columns. But beyond that it's laissez faire.
Cheeeeeeers 😁
@grizhlieCodesPosted almost 3 years agoOh yes - I'll probably correct minor styling things shortly. I seem to have omitted quite few 'details'.
0 - @BenjaDotMinSubmitted almost 3 years ago
Hello all! Today I learned about managing global state, using React and Redux. As always, pointers are welcome!
Thanks very much.
@grizhlieCodesPosted almost 3 years agoAwesome stuff man, love how close you got with the final result. Must have been satisfying when you saw that generated screenshot comparison 😁
I don't have much feedback in terms of code but here's an idea regarding accessibility:
- If you click on the cart you currently have to click on it again to close it.
- Implement a listener + function that closes the checkout when the user clicks the 'Escape' key.
- Also if the user clicks on anything that is not the cart, whilst the cart is open. Easy to do with another global listener (perhaps one that's created and destroyed depending on cart state) and
closest
link.
A few pet peeves
-
header
is located inmain
, any reason for putting it in main instead of thebody
or rather the container that you work with in React? I use Sveltekit but looks like similar concepts apply, some div or whatnot that acts likebody
. -
I personally avoid using fixed heights. Any reason to use it for the
header
? I usually build any height I need based on the content + gaps + paddings etc. -
Did you build the carousel? Wondering why it doesn't just loop when you reach the last/first image and then continue clicking in the same direction. Eg.: If we're on the first image and click
previous
it doesn't go to the last image. I'm only asking because I recently tried building it from scratch along with the transitions etc 😅
Awesome job though. Managing global state is when you start seeing the possibilities I think, I sure did.
Marked as helpful2 - @Duyen-codesSubmitted almost 3 years ago
I really need help with the unsolved: -header gradient background, when adding the second background with hsl, the background pattern disappeared, but with rgba(in this case i use kind of purple, it shows) -JavaScript for toggling navbar menu, burger icon and arrow icon, the menu showed very quickly and disappears right after that. It doesn't stay. -phones image stretches the whole container in art section and I don't know why.
Please tell me what I did wrong! I know there are other minor details that need to be fixed but for now I think about the above. Thanks a lot!
@grizhlieCodesPosted almost 3 years agoReal nice solution so far apart a few minor things you already mentioned :)
I'll makes notes one by 1 and reply to this comment chain as I spot stuff 👍
Your
burger-icon
should be a button as it activates some JS and doesn't take the user anywhere in reality, instead it opens up a modal. Because it's currently a link it refreshes the page every time you click it, hence the menu showing up for 1 second. If you look at the browser icon on the top you'll see that the page is refreshing.Marked as helpful0 - @Duyen-codesSubmitted almost 3 years ago
One thing I haven't figured out is the footer on desktop mode becomes have its own space somehow. Been sitting on this problem for more than an hour. I'd be grateful if you can have a look at my codes and direct me to the right path. Thanks a lot!
@grizhlieCodesPosted almost 3 years agoNice solution!
I'm not sure I understand the issue exactly, the footer looks fine to me unless I'm missing something obvious 😅.
What do you mean by 'own space'?
Marked as helpful0 - @Duyen-codesSubmitted almost 3 years ago
I hardcoded the data since I got stuck at injecting data from JSON file. Would really appreciate if you can give some tips on this problem. Thanks a lot.
@grizhlieCodesPosted almost 3 years agoDone. Link
I was editing on a new computer and new software so took longer than expected and is far from perfect imo - kept on hitting my head against sound issues 😒.
I think I covered the main gists you were looking for. I kept the JS very minimal and avoided some refactoring, for example fetching the data like Naser suggested. I did that initially then realised I'd be getting into the world of fetch and promises and thought otherwise to keep this beginner friendly.
I'd love any feedback/critique within YouTube if you end up watching it. You can find the JS bit within the video chapters.
0 - @Duyen-codesSubmitted almost 3 years ago
I hardcoded the data since I got stuck at injecting data from JSON file. Would really appreciate if you can give some tips on this problem. Thanks a lot.
@grizhlieCodesPosted almost 3 years agoJust built and uploaded my solution for this. Video will probably be made by tomorrow's end, will do most of it today evening. Sunday latest..?
Marked as helpful0 - @PrajwalDhuleSubmitted almost 3 years ago@grizhlieCodesPosted almost 3 years ago
Hi Prajwal,
Great attempt! As the most solid piece of advice, straight from the get-go:
Avoid using height. If you want to use
height
I recommendmin-height
. Why? GREAT QUESTION! The concept here is simple: we want to let the content decide how large a container is. When we write CSS we are working with alot of unknowns: What is the screen size of the user's client? What browser and what version are they using? Do they prefer darkmode or default? Etc.This gets into the core of FRONT END web development: we don't want to create pixel-perfect styling for the content. We want to create adaptable content that is responsive.
I actually made a video doing this project that will do a better job of sharing 'good approaches' (imo). Here's the link in case you're interested.
Feel free to bombard me with questions, I love this stuff :)
Cheers!
0 - @Duyen-codesSubmitted almost 3 years ago@grizhlieCodesPosted almost 3 years ago
Nice solution Duyen :), I like the use of grid!
A few shortcuts/tips:
When you want to declare a grid that will only have 1 row and 1 column AND will center its' child/ren all you need to do is add this code to the parent:
.grid-container { display: grid; place-items: center; }
That will do it. So I'm referring to your
.banner-overlay
for that. Oh yeah, if you inspect your code you will see that yourgrid-template-columns
andgrid-template-rows
are actually not working. You can't declare just1
but you can declare1fr
😁, then they would work fine. I think I also saw this mistake on.banner
.I'd also look into using
flex
withflex-flow: column nowrap;
andgap
to streamline stuff a little bit. I see that some of your elements have paddings/margins etc. I usually run a css-reset file that removes all default styling (especially margins/paddings) from ALL elements. Then I write them fresh.Overall awesome job, I assume you wanted to improve in
grid
a bit with this project? Love using it personally, it gives you such a controlled 'environment' to position elements with.Oh ya - I recorded me doing this solution, in case you want to check out what I might have done differently: link, only sharing because I think it might be beneficial, idc about 'views' as much (yet).
0 - @Drago78624Submitted almost 3 years ago
Here's my first FrontendMentor project completed by using Flexbox mainly Please let me know how can i improve, i always have a thought that im doing something unnecessary here and there😅
@grizhlieCodesPosted almost 3 years agoHello!
I know how you feel with 'doing something unnecessary'. That was at the forefront of my mind when I started coding and didn't know what I didn't know 😁
I started writing a bunch of stuff but I thought it'd be easier to just show you. So I made a short video, hope it's helpful.
I did also make a video recently of me doing the entire challenge (with SCSS, not sure if you're familiar with it) if you're interested: link.
1