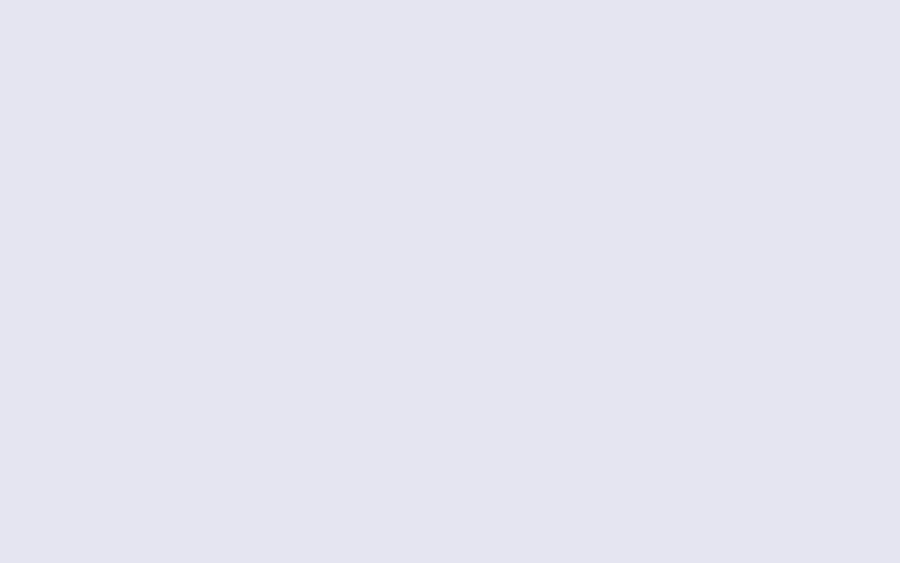
Design comparison
Solution retrospective
Drag and Drop Feature
I am developing using React and the @dnd-kit library for drag-and-drop functionality. I am encountering an issue where the event handlers for the checkbox (to check/uncheck items) and the delete button do not work after implementing the drag-and-drop feature. The checkbox does not toggle, and items cannot be deleted from the list. You can view the complete code in the following GitHub repository:
Is there something wrong with how I implemented @dnd-kit that is causing the event handlers to malfunction? How can I fix it so that the checkbox and delete functionality work after implementing drag-and-drop?
Community feedback
- @MunibAhmad-devPosted 5 months ago
Check Event Bubbling and Propagation:
Ensure that the drag-and-drop events are not stopping the propagation of click events needed by the checkboxes and delete buttons. Inspect the Drag Overlay:
Verify if the elements you are dragging have any overlay that might be blocking interactions with the checkboxes and delete buttons. Debug Event Listeners:
Use console.log statements or debugging tools to ensure that the event listeners for the checkboxes and delete buttons are still being attached correctly after implementing drag-and-drop. Prioritize Event Handling:
Make sure the order of event handling does not interfere with the drag-and-drop functionality. Example Code Here is an example demonstrating how you might structure your component to ensure proper handling of both drag-and-drop and click events: import React, { useState } from 'react'; import { DndContext, useDraggable, useDroppable } from '@dnd-kit/core';
const DraggableItem = ({ id, onDelete, onToggle, checked }) => { const { attributes, listeners, setNodeRef } = useDraggable({ id, });
return ( <div ref={setNodeRef} {...attributes} {...listeners}> <input type="checkbox" checked={checked} onChange={() => onToggle(id)} onClick={(e) => e.stopPropagation()} /> <button onClick={(e) => { e.stopPropagation(); onDelete(id); }} > Delete </button> <span>{id}</span> </div> ); };
const DroppableArea = ({ children }) => { const { setNodeRef } = useDroppable({ id: 'droppable', });
return <div ref={setNodeRef}>{children}</div>; };
const App = () => { const [items, setItems] = useState([ { id: 'item-1', checked: false }, { id: 'item-2', checked: false }, // Add more items as needed ]);
const handleToggle = (id) => { setItems((prevItems) => prevItems.map((item) => item.id === id ? { ...item, checked: !item.checked } : item ) ); };
const handleDelete = (id) => { setItems((prevItems) => prevItems.filter((item) => item.id !== id)); };
return ( <DndContext> <DroppableArea> {items.map((item) => ( <DraggableItem key={item.id} id={item.id} onDelete={handleDelete} onToggle={handleToggle} checked={item.checked} /> ))} </DroppableArea> </DndContext> ); };
export default App; "
Stopping Event Propagation:
For the checkbox and delete button, e.stopPropagation() is used to prevent the drag-and-drop functionality from interfering with the click events. Use of DndContext:
The DndContext provides the necessary context for the drag-and-drop functionality. Separating Concerns:
The DraggableItem component is responsible for handling its own drag-and-drop, click, and change events, ensuring that these do not interfere with each other. State Management:
State is managed in the App component, and helper functions (handleToggle and handleDelete) are passed down to manage the state changes.
Marked as helpful0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord