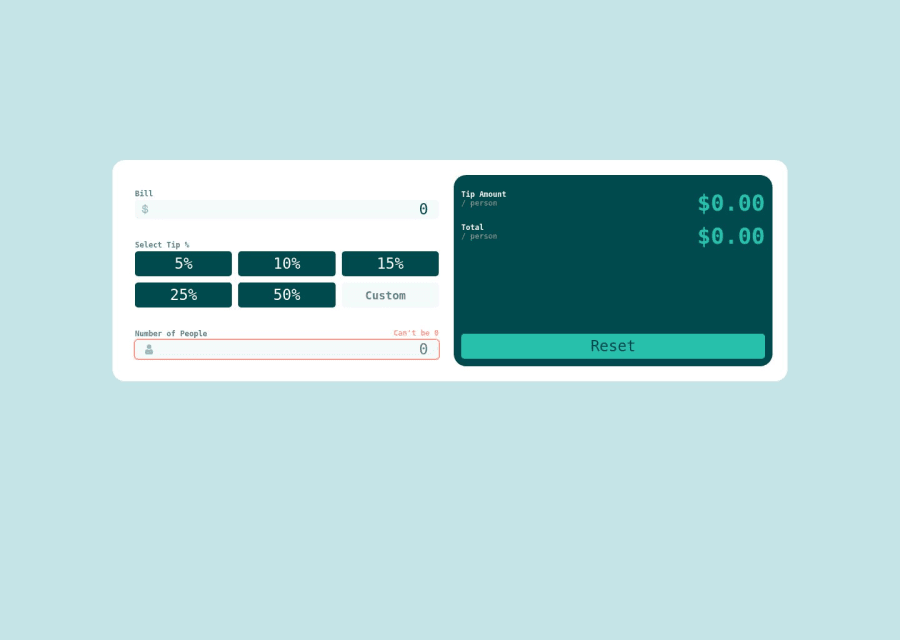
Design comparison
SolutionDesign
Solution retrospective
I have been stuck in tutorial hell for the last few months, so this is my very first attempt at creating something completely on my own. Feel free to be as brutally honest with my work.
Difficulties:
- The SVG icons in the input fields. I converted the SVG into a React component and used CSS position property to position inside the input field. This took me forever to figure out, and I'm wondering if there's an easier way to accomplish this.
- Styling the "/ person" to drop below the "Tip Amount" and "Total" lines without pushing the dollar amounts down with it. This was extraordinarily difficult for me. I ended up making each label into a flexbox, direction: column, and wrapped the dollar amount to the next column.
- Some of the React logic is probably not efficient. The reset button was specifically challenging to figure out.
Best Practices:
- React file organization. I'm sure I should organize my React components into separate folders, but I'm not sure of React component organization best practices here.
- My App component file seems very bloated. I think I've got everything working here, but I've seen some React App component files that are much leaner than mine is. I'm wondering if mine is too bloated and some of the logic should be moved to other components?
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord