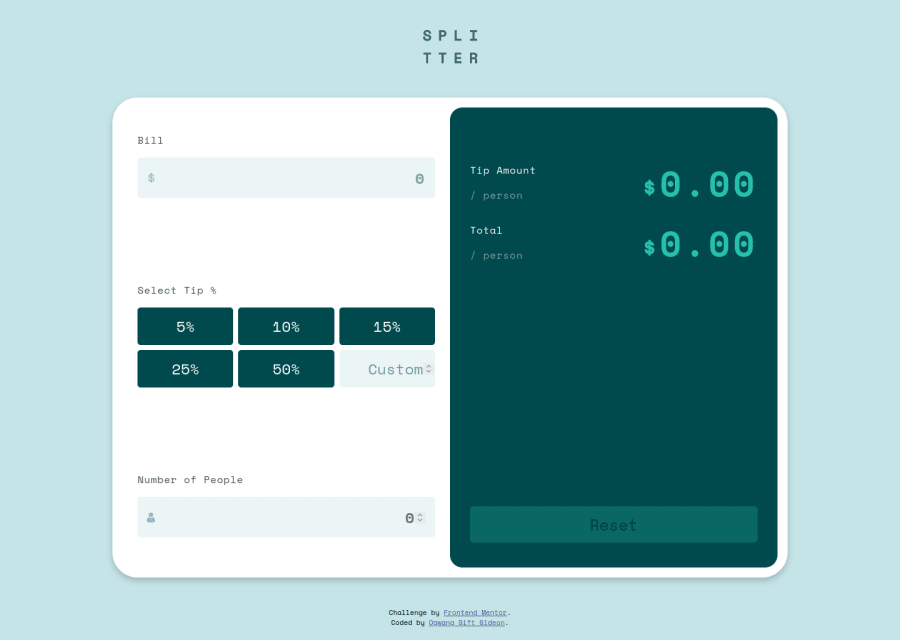
Design comparison
Solution retrospective
I would like to get some guidence on how to program a complex multi-functional calculator
Community feedback
- @AlexKMarshallPosted about 3 years ago
In your script file you're setting an initial value for costPerPerson as 28.51. This means if you just press a tip percent button, and don't enter a bill total, or the number of people, you're still showing a calculated value, which doesn't make sense.
One way to structure this will be to have a state object, which would look something like
const inputState = { bill: 0, tipPercent: 0, people: 0, }
You can have handlers for when someone enters an input or selects a tip percent that updates the values in that input state.
Then you have a separate function to render the results. Something along the lines of
function isInputValid() { return inputState.bill > 0 && inputState.tipPercent > 0 && inputState.people > 0 } function calculateOutput() { if (!isInputValid) { return {tipPerPerson: 0, totalPerPerson: 0, reset: 'disabled'} } return { tipPerPerson: (inputState.bill * inputState.tipPercent) / inputState.people, totalPerPerson: (inputState.bill * (1 + inputState.tipPercent)) /inputState.people, reset: 'enabled' } } function renderOutput() { const output = calculateOutput() elTipAmount.innerText = output.tipPerPerson elTotalAmount.innerText = output.totalPerPerson if (output.reset === 'enabled') { elResetButton.removeAttribute(''disabled") } else { elResetButton.setAttribute("disabled") } }
Then you'd call that renderOutput function in all of your change handlers, which keeps your outputs in sync with your inputs.
There's plenty of other ways to do it too, but I think that one keeps the logic nice and tidy, makes it relatively easy to add field and form level validation logic just by modifying one of the sub functions.
Marked as helpful0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord