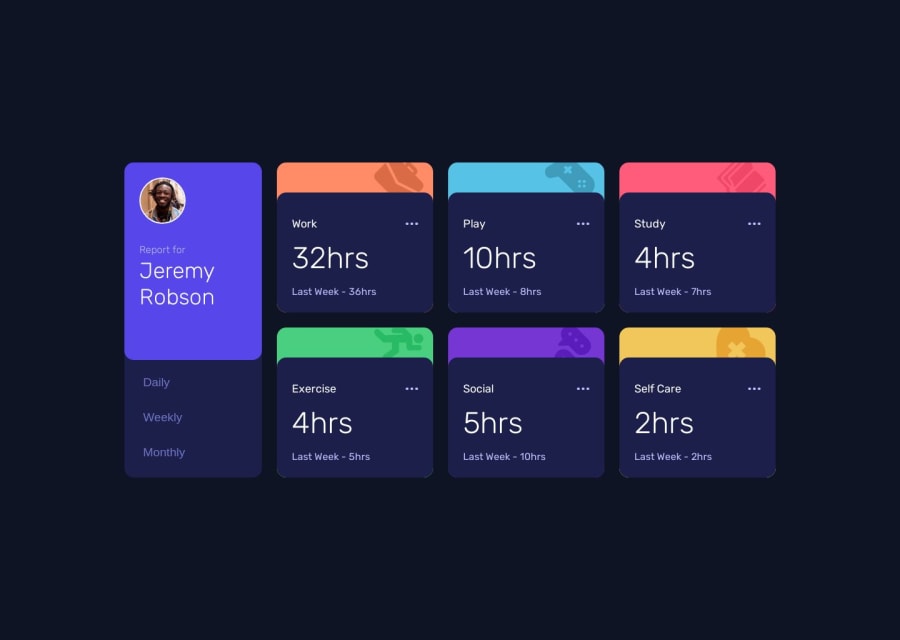
Design comparison
Solution retrospective
The almost perfect design was not planned. x_x
Since I stopped coding with React for a while I'm struggling a bit with hooks and components but that ain't something that would stop me though.
Community feedback
- @Remy349Posted 11 months ago
Hi @amrmabdelazeem, many congratulations on completing the challenge, it is really amazing and the final design is great.
I reviewed your React code and I want to give you a tip to refactor the code found in the App.jsx component. I cloned your repository and edited the code to change the logic you have when rendering the Activity.jsx component. Instead of doing those component renderings using the if else you have nested, I recommend this way:
import React, { useState } from "react"; import Heading from "./Heading"; import Activity from "./Activity"; import data from "../data.json"; export default function App() { const [time, setTime] = useState("weekly"); function handleTime(childTime) { setTime(childTime); } const getTimeframeData = (item, timeframe) => ({ key: timeframe, current: item.timeframes[timeframe].current, previous: item.timeframes[timeframe].previous, }); return ( <div className="container"> <Heading onHandle={handleTime} /> {data.map((item, index) => { const timeframeData = getTimeframeData(item, time); return ( <Activity key={index} id={index} title={item.title} current={timeframeData.current} previous={timeframeData.previous} /> ); })} </div> ); }
A little explanation:
Function getTimeframeData:
const getTimeframeData = (item, timeframe) => ({ key: timeframe, current: item.timeframes[timeframe].current, previous: item.timeframes[timeframe].previous, });
- This function takes two arguments: item (representing an element of the array data.json) and timeframe (representing the current time period).
- Returns an object with the keys key, current and previous, which correspond to the specific properties of the time period within the item.
Component Rendering:
return ( <div className="container"> <Heading onHandle={handleTime} /> {data.map((item, index) => { const timeframeData = getTimeframeData(item, time); return ( <Activity key={index} id={index} title={item.title} current={timeframeData.current} previous={timeframeData.previous} /> ); })} </div> );
- Then, a mapping is performed on the array data using the map method.
- For each item element, getTimeframeData is called to obtain the data specific to the current time period (time) and an <Activity /> component is rendered with that data.
In short, the code uses the time state to track the current time period and a getTimeframeData function to get the time period specific data for each activity in the data array. Then, the interface is rendered based on this data.
CONGRATULATIONS ON COMPLETING THE CHALLENGE. I hope my advice will be of great help to you :)
Marked as helpful0@amrmabdelazeemPosted 11 months ago@Remy349 Thank you for the heads up! I've been meaning to working on my refactoring skills over this one, but you really gave me ideas on how to tackle it!
0@Moulaye-dagnonPosted 11 months ago@Remy349 Hi! I completed this challenge too and for your tip I have one question . Is it neccessary to add {Key} in the function getTimeframeData . Great work @amrmabdelazeem
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord