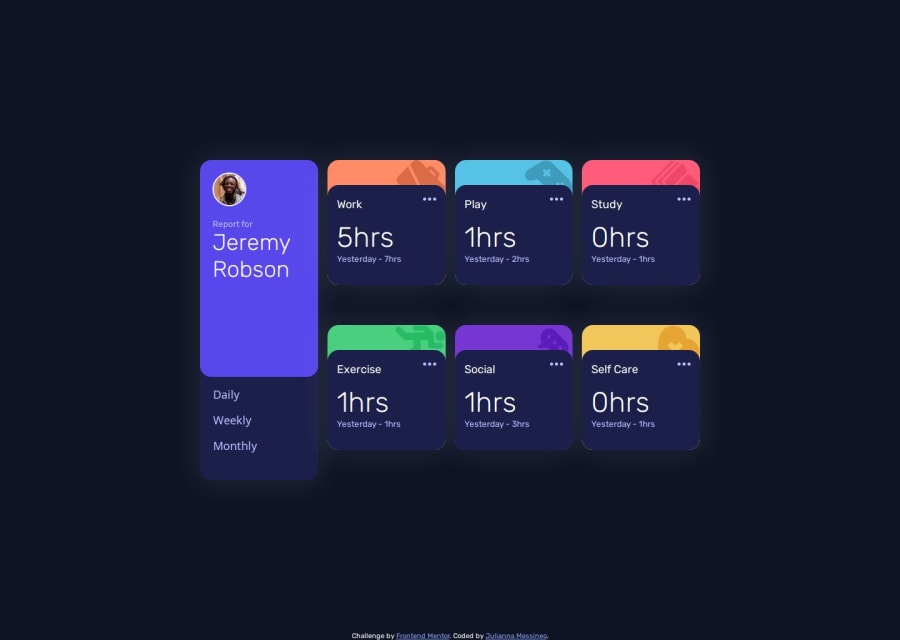
Design comparison
Solution retrospective
I'm proud of fetching and using the data from the JSON file. I needed some help to figure it out, and I'm not sure I could do it again without looking at what I did, but I understand it a little better. I'm also proud of how my website was easily responsive to different screen sizes with this line of code on my container in CSS
grid-template-columns: repeat(auto-fit, minmax(180px, 1fr));
I was having issues with fetching and then using the data before it was fully fetched. I had to get help and learn where I could put my function within the .then function so that it wouldn't run until the data has been fetched.
What specific areas of your project would you like help with?Chat GPT gave me the suggestion to use this code:
fetch("./data.json")
.then((res) => res.json())
.then((data) => {
timeData = data; // Assign fetched data to timeData
fetchTimeDaily(timeData); // Call your function with timeData after it's fetched
})
.catch((error) => {
console.error('Error fetching data:', error);
// Handle errors here
});
and it fixed all of my problems but I'm still not completely understanding why. Wouldn't the computer try to execute fetchTimeDaily(timeData) before timeData is set equal to data if this takes a little longer and they are both inside of the same .then statement?
Would it be better practice to do
.then((data) => {
timeData = data; // Assign fetched data to timeData
}
.then (not sure what syntax goes here??)
fetchTimeDaily(timeData);
})
to make sure it waits until the first line has been fully executed?
Community feedback
- @dylan-dot-cPosted 5 months ago
Hey Julianna! This is an interesting solution and you did well, I like how you added the popup menu to the cards when you click them.
Firstly for your question... normally fetch is like this
fetch('./data.json') //gets file from storage .then(res => res.json()) //turns the file into a json object .then(data => { timeData = data; //set data to your global value timeData fetchTimeDaily(timeData)//use your object to get the daily data }) .catch(e) .....
so you can essentially call
fetchTimeDaily(data)
before doingtimeData = data;
this isn't really bad but maybe the function uses timeData as the global variable and not the timeData parameter and maybe that could cause problems.So the code you provided isn't correct as the data.json file needs to be fetched first then turned into json and then and only then can you use it in your code... hoope all of that is understandable.
Here are a few ways to improve your code!
- Layout: You have used grid and
grid-template-columns: repeat(auto-fit, minmax(180px, 1fr));
to make it responsive which is good, but you might need to do a few more stuff to control the width and height of the grid. You also hadposition:absolute
on the grid container but you could use better ways to center it. - Accessibility: You used buttons which is good but you need to use more semantic html tags like
main
,article
,section
and others instead of overusing divs in your code. Which can help screen-readers to understand your website better. - Fixed WIdths and Heights: Some places you have these and if you used them as %s it becomes hard to control the height of the containers.
- Not using DRY code: Your JS code solves the problem but it's heavily repeated! You can break up your code into smaller functions and make use of arrays and dom Manipulation functions like
querySelectorAll
to loop through the arrays and only do it once and not 6 times. Code like this is hard to debug and change in the future. Same goes for the 3 buttons as that could be one function... let me give you a example
<button value="daily" class="timeframe--button">Daily</button> <button value="weekly" class="timeframe--button">Weekly</button> <button value="monthly" class="timeframe--button">Monthly</button>
const buttons = document.querySelectorAll('.timeframe--button'); buttons.forEach( btn => { btn.addEventListener("click", e => { let data = btn.value; timeframe = data; updateData(timeframe); }) })
You should have an updateData function that is smart enough that it takes the current timeframe and updates the info using that data.
This is alot but its important for being a better programmer.
You could also check out my solution to see how I did it as I also used vanilla js for mine.
Take care and have a great day!
Marked as helpful0@mathematiCodePosted 5 months ago@dylan-dot-c Thank you! This is very helpful. I really appreciate the focus on writer cleaner code as that's a big focus for me.
1 - Layout: You have used grid and
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord