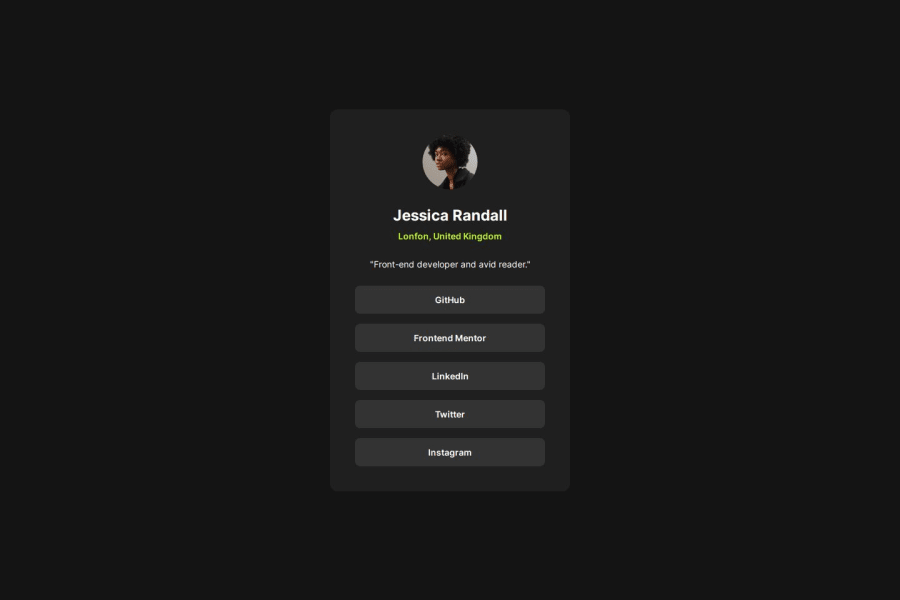
Responsive Social Links Profile using HTML, CSS, and JavaScript
Design comparison
Solution retrospective
Any feedback would be very helpful. Thanks!
Community feedback
- @MuhammadFarhanRosidiPosted about 2 months ago
JavaScript (
script.js
) - Suggestions for Improvement:-
Avoid querying the DOM repeatedly:
- The
cardContainer
anddustContainer
elements are being queried inside themousemove
event listener on every movement. Since these elements do not change, it's better to query them once and store them outside the event listener.
Improvement:
const cardContainer = document.querySelector('.card-container'); const dustContainer = document.querySelector('.dust-container'); document.addEventListener('mousemove', (e) => { if (!cardContainer.contains(e.target)) { createDust(e); } }); function createDust(e) { const dust = document.createElement('div'); dust.classList.add('dust'); dust.style.left = `${e.pageX - 4}px`; dust.style.top = `${e.pageY - 4}px`; dustContainer.appendChild(dust); setTimeout(() => dust.remove(), 500); }
This approach improves performance by avoiding repeated DOM queries.
- The
-
Refactor the dust creation process:
- The dust creation logic can be separated into a dedicated function (as shown in the above improvement) to make the code more modular and reusable.
-
Check if
dustContainer
exists:- Before appending dust, make sure the
dustContainer
is available, especially if it is dynamically created or removed.
- Before appending dust, make sure the
CSS (
styles.css
) - Suggestions for Improvement:-
Use
rem
orem
for consistent spacing:- Instead of using fixed pixel values like
px
, it’s a good practice to userem
orem
for margins, padding, and font sizes. This ensures better responsiveness and scalability across different screen sizes.
Improvement:
h1 { font-size: 1.5rem; /* Already using rem, which is good */ } .card-container { width: 24rem; /* 384px becomes 24rem */ padding: 2.5rem; /* 40px becomes 2.5rem */ }
- Instead of using fixed pixel values like
-
Consider adding a fallback for the
background-color
:- While you’ve defined a primary color palette with
:root
, it's still good to ensure fallback colors for broader compatibility.
Improvement:
.card-container { background-color: var(--medium-gray, #1F1F1F); /* Added fallback color */ }
- While you’ve defined a primary color palette with
-
Improve animation for
dust
trail:- The dust animation could be smoother by adjusting timing, easing functions, or adding keyframes for better visual appeal. For instance, slowing down the animation for a more lasting effect.
Improvement:
@keyframes dust-animation { 0% { transform: scale(1); opacity: 0.7; } 50% { transform: scale(2); opacity: 0.4; } 100% { transform: scale(3); opacity: 0; } }
-
Media Queries (Improve Responsiveness):
- Add more breakpoints for different screen sizes. Right now, it only has
768px
for tablets and375px
for small mobile devices. You could add more to make it more adaptable for larger tablets, phablets, etc.
Improvement:
/* For large tablets */ @media screen and (max-width: 1024px) { .card-container { width: 30rem; /* 480px */ } } /* For smaller devices */ @media screen and (max-width: 480px) { .card-container { width: 100%; /* Full width on very small screens */ } }
- Add more breakpoints for different screen sizes. Right now, it only has
-
Dark Mode Considerations:
- If you want to extend the design to support a dark mode toggle, consider using CSS custom properties (variables) that can be switched dynamically. This would make the site more versatile for different user preferences.
General Improvement Suggestions:
-
Performance optimization: Using
requestAnimationFrame
for better handling of themousemove
event could help optimize performance, especially if the mouse trail becomes more complex.Example:
let lastTime = 0; document.addEventListener('mousemove', (e) => { const currentTime = Date.now(); if (currentTime - lastTime >= 16) { // approx 60 FPS if (!cardContainer.contains(e.target)) { createDust(e); } lastTime = currentTime; } });
-
Accessibility considerations: Ensure your links have meaningful, accessible content (for screen readers), such as using
aria-label
for social media links to make it more inclusive for users with disabilities.
Marked as helpful0 -
- @0maaPosted about 2 months ago
A very nice design. Looks almost like the given project, well done!
0 - @AndersonPauloPosted about 2 months ago
Compared to other codes I've seen, this one stands out for its quality and performance." Code optimization is above average, which demonstrates special care for performance.
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord