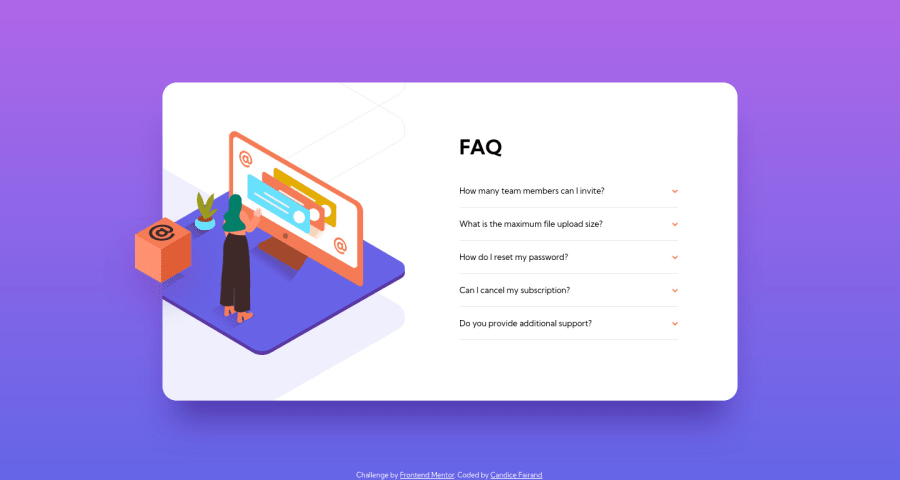
Submitted almost 3 years ago
Responsive FAQ component using Flexbox and relative positioning
#sass/scss
@Candyfair
Design comparison
SolutionDesign
Solution retrospective
I really struggled with the positioning of the images for the desktop layout, and finally understood that I had to put them in divs to position them more easily.
Also, I couldn't find a way to close all the other answers when one question is clicked AND still be able to close the opened answer by clicking again on the question. Does anyone have an idea of the way it should be implemented? Thanks!
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord