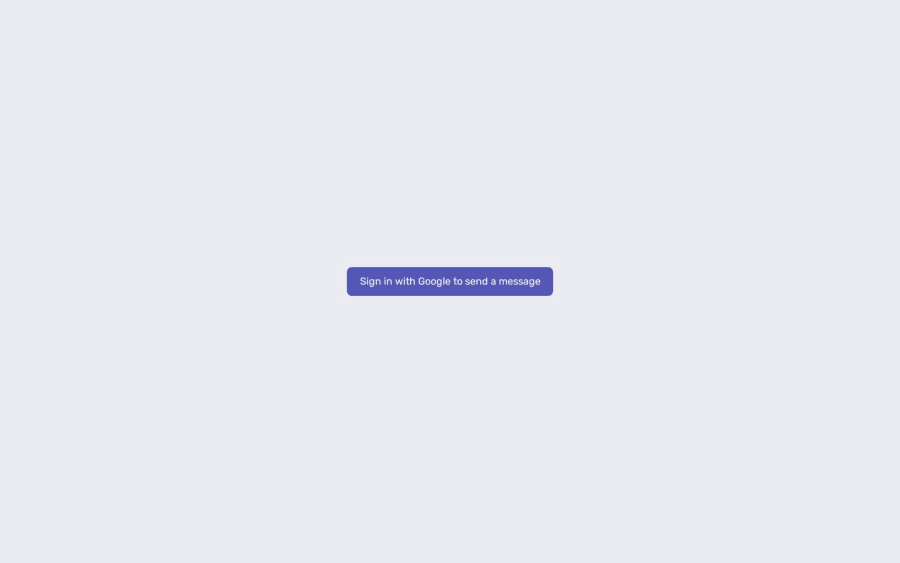
Real-time and partly CRUD interactive comment section
Design comparison
Solution retrospective
I wrote my answers in the comments, but again, any feedback is appreciated.
Community feedback
- P@huyphan2210Posted 4 months ago
Hi, @KapteynUniverse
Great work on this challenge! I checked out your solution and I have some thoughts:
- Adding a loading screen: With your current setup, you can manage the loading state by using MobX, a state management library. Here's how you can implement it:
- Create a
LoadingStore
:
// LoadingStore.js import { makeAutoObservable } from "mobx"; class LoadingStore { isLoading = true; constructor() { makeAutoObservable(this); } setLoading(loading) { this.isLoading = loading; } } export default new LoadingStore();
- Update
getDoc.js
to use MobX for loading:
// getDoc.js - consider to use "useComments.js" for consistency import { useEffect, useState } from "react"; import loadingStore from "./LoadingStore"; // Import MobX store const useComments = () => { const [comments, setComments] = useState([]); useEffect(() => { // Fetch data... loadingStore.setLoading(true); // Set loading to true initially const unsubscribeComments = onSnapshot(query(...), (querySnapshot) => { setComments(commentsData); loadingStore.setLoading(false); // Set loading to false after fetching }); return () => unsubscribeComments(); }, []); return comments; };
- Use
isLoading
in yourHome
component:
// Home.jsx import { observer } from "mobx-react-lite"; // Import the observer HOC from MobX to make the component reactive import loadingStore from "./LoadingStore"; // Import the MobX store that holds the loading state const Home = observer(() => { // If loading is true, show the loading screen if (loadingStore.isLoading) return <div className="loading-screen"></div>; // Once loading is false, render the content (for example, "Content Loaded") return <div>Content Loaded</div>; }); export default Home;
This should make the
isLoading
state globally accessible and simplifies handling loading logic.Let me know what you think!
Marked as helpful0@KapteynUniversePosted 4 months agoHey @huyphan2210, Thanks for the feedback. Before submitting the solution for the issue with comments jumping on page load, I tried creating a custom loading component without using a package. I added it to the App.jsx, used some useState and useEffect to check if the home page had loaded, and then displayed the home page once it was ready. But the comments still kept jumping, so I removed the loading page because it was just increasing wait/load time.
I’ve tried your suggestion on local files too, and it looks like it’s working in combination with the fade-in animation, which was one of the other leftover fixes I had tried. I’ll explore it more later. Thanks again!
1 - @KapteynUniversePosted 4 months ago
I don’t even have an animation, don't do this to me screenshot :D That reminds me (I know why the screenshot looks like that, but I don’t know the reason for that issue). I tried to add a loading screen to fix it, but the comments still jumped after the loading screen.
1@dylan-dot-cPosted 4 months ago@KapteynUniverse interesting, maybe it depends on how fast the website gets the data before fem takes a screenshot, the thing is that maybe NEXTJS and SSR would make it faster but you can't have state in server components and that would make it harder to use ig.
1 - @MahmoodHashemPosted 4 months ago0@KapteynUniversePosted 4 months ago
Hey @MahmoodHashem, thank you. I am not an expert and it is a bit late for me but i will check your code tomorrow and give feedback if i can.
To answer your question: You need a database. I used Firebase for the real time database and authentication.
1@MahmoodHashemPosted 4 months ago@KapteynUniverse Alright, take your time, brother.
I’ve heard about Firebase before and even followed a tutorial to create a notes app with it. However, I didn’t realize it could be used for this kind of functionality. I’ll look into it, and if it seems simple enough, I’ll implement it in my project.
0@KapteynUniversePosted 4 months agoHey @MahmoodHashem, i checked your solution. I don't have much feedback to give tbh. I am very new to React but the issue you are having with the replies feels like a mapping issue. Maybe tracking the user with a useEffect hook and update on the replies might fix the issue.
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord