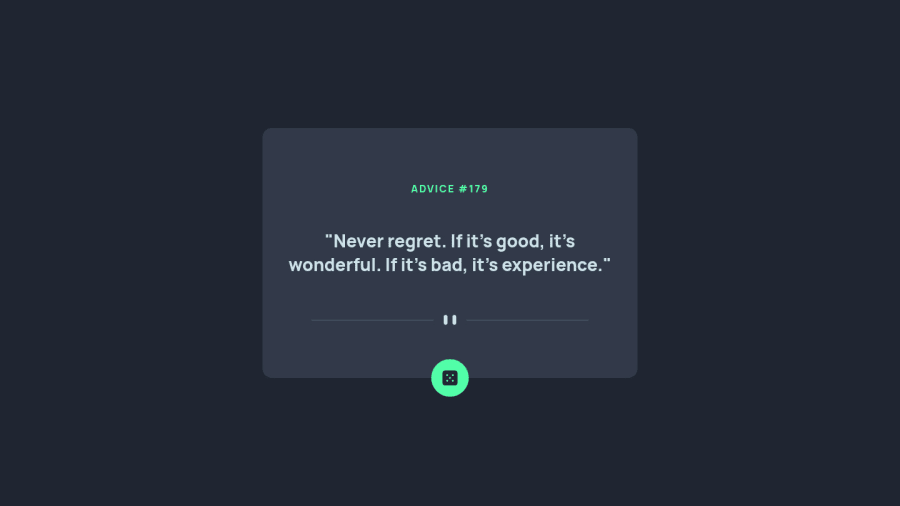
Random Advice Generator using React and SCSS
Design comparison
Solution retrospective
Hi all,
I really enjoyed this challenge! This was my first time attempt using async await with an API call, as well as useEffect. Any feedback on my async function is welcome!
const getData = async () => {
try{
const res = await fetch('https://api.adviceslip.com/advice')
const data = await res.json()
setAdvice(data.slip.advice)
setAdviceNum(data.slip.id)
} catch (error){
console.error(error)
}
}
Thanks! -Tyler
Community feedback
- @Ibarra11Posted over 2 years ago
One improvement you can make with your useEffect is to add an empty dependency list. As of right now, getData is getting called twice. When you click on the advice button, it calls the getData function, which then calls setState and React is going to queue a re-render. After React renders with the new state, it checks the useEffect and since you don't have any dependencies, React is going to run the useEffect after every render, which causes getData to get called again. In this case, you only want the useEffect to run on the initial render to get the first advice and from that point forward the action of getting new advice data should come from clicking the button.
useEffect(() =>{ getData() }, [])
Marked as helpful3@tylermaksPosted over 2 years ago@Ibarra11 thanks so much! I didn't realize that useEffect was called after each render, I'll definitely keep this mind. I've updated my code based on your comments and things are working smoothly - thanks again!
-Tyler
0@elaineleungPosted over 2 years ago@Ibarra11 Glad you mentioned the dependency array because I also meant to as well 🙂
0 - @elaineleungPosted over 2 years ago
Hi Tyler, the app looks good! As @shivaprakash-sudo said, on Firefox the advice only either loads on the first load and then doesn't load, or it would need a few seconds for the next load despite clicking on the button. I solved this problem by adding a cache object in fetch:
fetch("https://api.adviceslip.com/advice", { cache: 'no-cache' })
If you have Firefox, try it out and see.
Marked as helpful1 - @shivaprakash-sudoPosted over 2 years ago
Hello Tyler,
The code looks fine to me.
Side note: I don't know why, but the app doesn't seem to work in my Firefox, it works on other browsers, but even in them, I have to wait for 2 or 3 seconds to click the button for new advice, since clicking the button immediately doesn't seem to work. I tested the code too on my machine and the same thing happens, for some reason. Has this ever happened to you?
Marked as helpful1@elaineleungPosted over 2 years ago@shivaprakash-sudo I have the same issue on Firefox!
1@tylermaksPosted over 2 years ago@shivaprakash-sudo thank you for catching this! I've made some updates based on comments from @elaineleung and it fixed the issue with Firefox and the delay between the click and the new advice.
Thank you so much! -Tyler
1@shivaprakash-sudoPosted over 2 years ago@tylermaksymiw
It's working perfectly now. Good work, Tyler.
0 - @0-BSCodePosted over 2 years ago
Awesome work, Tyler! Your async await code looks good. For future projects, I would suggest getting into organizing your React project's file structure. You can read more about it here. Although for simple projects like this one it may not be needed, I think it's a good idea to get into the habit of organizing your files early on so that when you eventually get into large projects it's easier to structure them.
Keep up the good work! Cheers🎉
Marked as helpful0@tylermaksPosted over 2 years ago@0-BSCode I hadn't considered this until now. This is really helpful, thank you!
- Tyler
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord