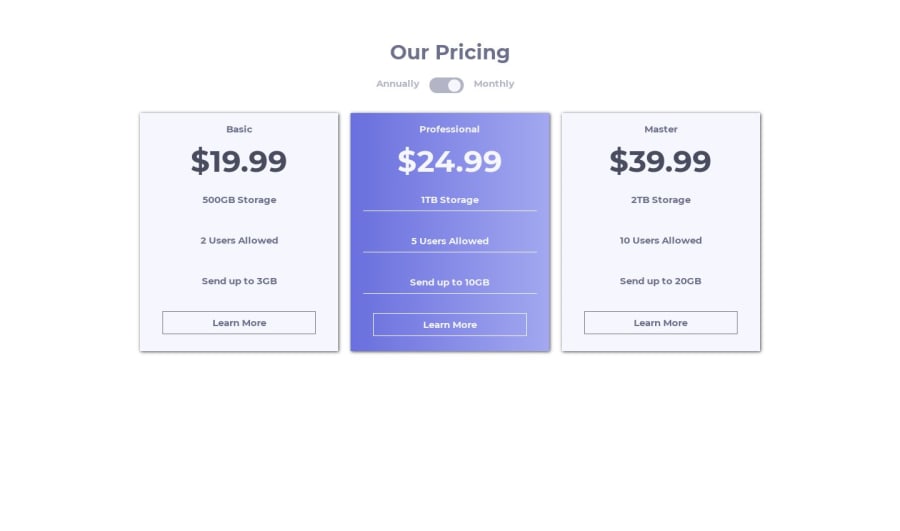
Submitted over 4 years ago
Pricing component with toggle with SASS
@alejatraveler98
Design comparison
SolutionDesign
Solution retrospective
Is there another way to make the price change using Arrays?
Community feedback
- Account deleted
Hi.
There's a very scalable way to do this and I'll explain it in simple steps.
- Create a global variable to store the switch element:
const toggle = document.querySelector('#toggle');
- Create an object to define the plans:
const plans = { monthly: { basic: '19.99', pro: '24.99', master: '39.99', }, annually: { basic: '199.99', pro: '249.99', master: '399.99', }, };
- Add a data attribute in the tags where you'll show the prices and leave its content empty
<div class="cards-items__pricing" data-plan="basic"></div> ... <div class="cards-items__pricing" data-plan="pro"></div> ... <div class="cards-items__pricing" data-plan="master"></div>
- Create a function to handle the plan change
const handlePlanChange = e => { const checked = toggle.checked; // Get elements by data attribute const priceElements = document.querySelectorAll(`[data-plan]`); // 1. Loops between the tags that have the data-attribute. // 2. Gets the type (monthly | annually) depending on if the switch is checked // 3. Get the value of the data attribute // 4. Remember the 'plans' object? Here we access the plan using bracket notation // 1 for (const p of priceElements) { // 2 const type = checked ? 'monthly' : 'annually'; // 3 const plan = p.getAttribute('data-plan'); // 4 p.innerHTML = plans[type][plan]; } };
- Add event listeners to call the above function on load and on switch's value change
window.addEventListener('load', handlePlanChange); toggle.addEventListener('change', handlePlanChange);
Let me know if this helps. Here is the full implementation
3@alejatraveler98Posted over 4 years ago@juanmesa2097 Thank you very much, I managed to implement it in my code, if it is a simpler but scalable way, it was a great help
1Account deleted@alejatraveler98 of course, I'm glad you managed to implement this solution on your code. 👍
1
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord