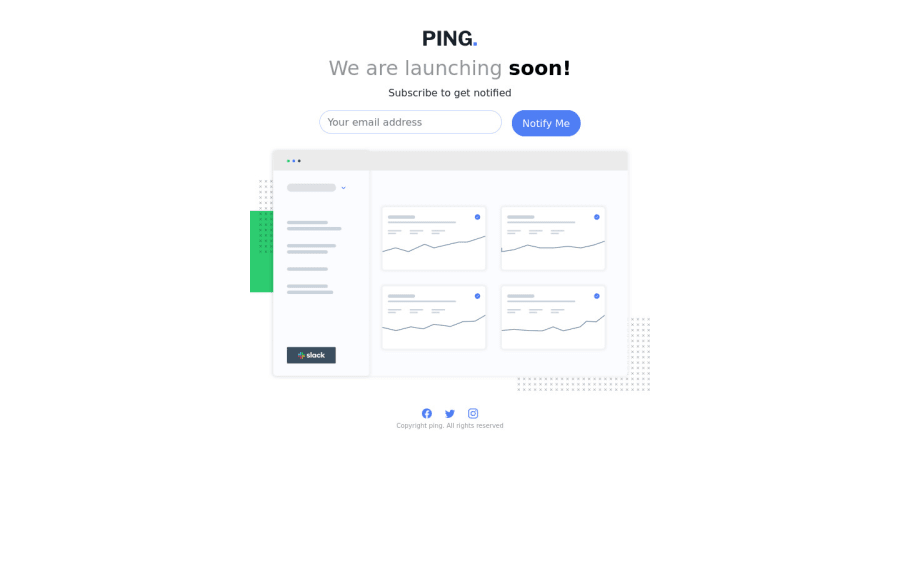
Ping coming soon page with html/css/bootstrap/JavaScript
Design comparison
Solution retrospective
Any idea why the regexp is not working
Community feedback
- @FluffyKasPosted over 2 years ago
Heyo,
Next time when you upload a solution, please provide a link to the repo in question and not to your profile, so people don't have to look for the relevant code.
Regarding the Javascript issue, this is where your problem most probably comes from:
let id = (id) => document.getElementById(id) let classes = (classes) => document.getElementsByClassName(classes) let email = id('email'), form = id('form'),
There are some Javascript basics missing here. I don't want to provide a solution for you just yet, as that's not the best way to learn. Instead, I'll give a hint: look up the MDN articles for getElementById and getElementsByClassName and see how they work exactly. Also look up how to declare variables and target html elements, see whether you did them correctly here. See if you got the basic syntax right.
If something isn't working, the best way to find the source of the problem is using console.log. Go step by step.Double check if the variable corresponds with the html element you wanted to target. Console log everything >.< Also make sure you have ESLint set up in your code editor. It catches a lot of the issues, like syntax errors and such.
0@Tessie475Posted over 2 years ago@FluffyKas thanks. Although the problem Is with the regex not the syntax. The first regex I used didn't match an email. I changed it and put one that matches but it still doesn't bring up an error when an invalid email Is inputted
0@FluffyKasPosted over 2 years ago@Tessie475
From what I understand: when you give your input a type="email", it provides some basic checks to see whether the value is a valid email. Since this doesn't cover all use cases, people also add a regex to it. For most email values you will try though this default mechanism provided by the input type will overwrite whatever javascript you wrote for this, so you'll only see your error styles if you try an email value that ISN'T covered by this default input mechanism.
What you could do (you may want to double-check this with someone more knowledgeable about accessibility but this is my best guess):
-
Remove the type="email" from the input. I wouldn't suggest this though, I guess this isn't great even though it technically works.
-
You can add a novalidate tag for the form that prevent all browser validation. I suppose this is a better option, if you trust your own validation rules enough.
You could also clean up your Javascript a bit! At first glance, I thought you're having syntax error problems but in reality it was just a bit hard to understand what's going on.
This is what I came up with and it seems to work fine (again, not considering accessibility, for now):
HTML:
<form id="form" class="row g-3 justify-content-center" novalidate> ...
JS:
const email = document.getElementById("email"); const form = document.getElementById("form"); const errMsg = document.querySelector(".error"); form.addEventListener('submit', (e) => { e.preventDefault(); engine(email.value, 'Please provide a valid email address') }) let engine = (emailValue, message) => { if (emailValue.trim() === '' || !emailValue.match(/^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/)) { errMsg.innerText = message; email.style.border = '2px solid red'; } else { errMsg.innerText = ''; email.style.border = '2px solid green'; } }
Targeting your variables like this will make your code more readable! Or just skip this if you're not interested in this and just add that bit of tag in your html I mentioned above.
Marked as helpful0 -
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord