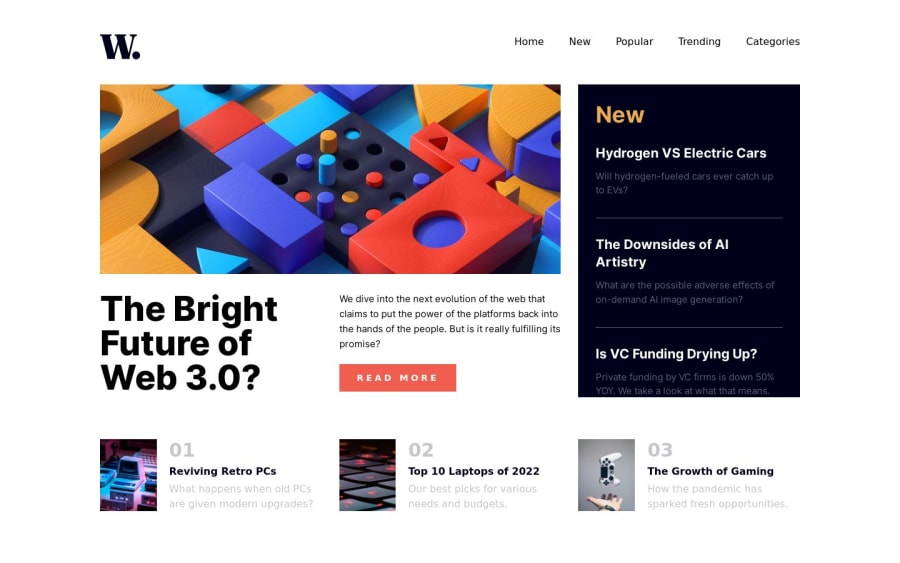
Design comparison
Solution retrospective
I was able to practice my React fundamentals and Tailwind CSS skills. The only issue is that when the hamburger icon is clicked and the menu items pop out, when you scroll further, it doesn't close. This is because I don't know how to apply the IntersectionObserver API in React. I have done some research, and I am seeing information concerning useEffect and useRef. When I learn them, I will update my code later. But if you know of a better way to make an element disappear when scrolled past using useState, let me know.
Something like this in normal javascript:
const initialCoords = section1.getBoundingClientRect();
window.addEventListener("scroll", function (e) {
if (this.window.scrollY > initialCoords.top) nav.classList.remove("sticky");
});
But in my code:
I want to setShowMenu(false)
when the menu item is scrolled past.
const [showMenu, setShowMenu] = useState(false);
{showMenu && (
<div className="absolute bg-offWhite min-h-screen w-[64%] right-0">
<div className="relative">
<img
className="md:hidden absolute right-7 top-3"
src={menuClose}
alt={"X diagram to close the menu"}
onClick={menuHandler}
/>
<div className="space-y-6 p-6 pt-28 text-xl">
{navItem.map((nav) => (
<NavItems nav={nav} key={nav.id} />
))}
</div>
</div>
</div>
)}
Thank you for your time and feedback. Have a nice weekends!
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord