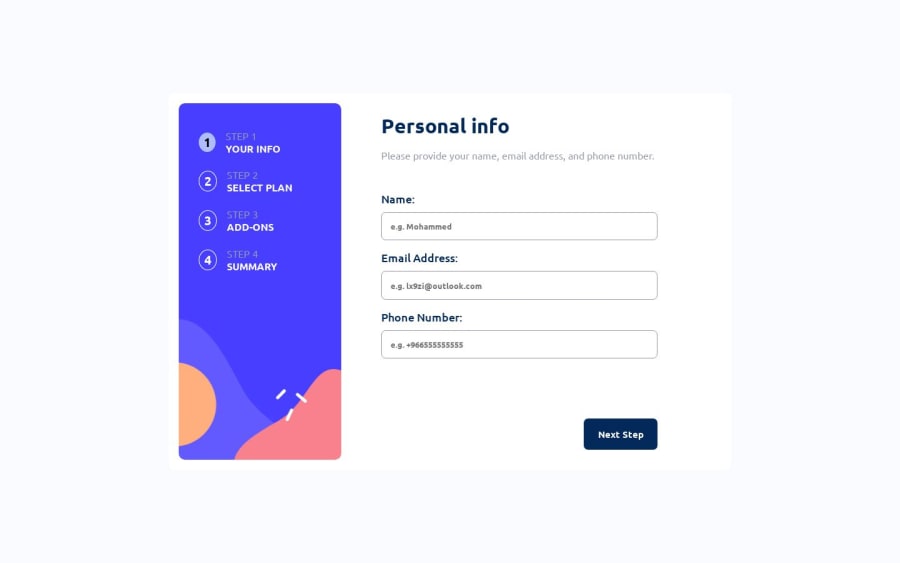
Design comparison
Community feedback
- @Saad-HishamPosted over 1 year ago
Hi there😀 I wanted to let you know that I think the design of your project is really great, and the transitions between steps are amazing as well. However, I noticed that there doesn't seem to be any validation in place. Don't worry though, there are multiple ways to add validation to your form!
One way to do this is by adding the required attribute to the input fields in your HTML code and using a submit button. This ensures that the user fills out all required fields before submitting the form. Here's an example:
<form method="POST" action="/submit-form"> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="number">Number:</label> <input type="tel" id="number" name="number" pattern="[0-9]{10}" required> <button type="submit">Submit</button> </form>
Validation is typically performed on the server side using backend technologies in most real-world projects. However, as front-end developers, we can also implement validation using JavaScript to provide real-time feedback to the user and enhance the user experience. Here's an example of how you can implement client-side validation using JavaScript:
const form = document.getElementById("myForm"); const submitBtn = document.getElementById("submitBtn"); form.addEventListener("submit", function(event) { event.preventDefault(); if (validateForm()) { form.submit(); } }); function validateForm() { let isValid = true; const name = document.getElementById("name"); const email = document.getElementById("email"); const number = document.getElementById("number"); if (name.value === "") { alert("Please enter your name."); isValid = false; } if (email.value === "") { alert("Please enter your email address."); isValid = false; } else if (!isValidEmail(email.value)) { alert("Please enter a valid email address."); isValid = false; } if (number.value === "") { alert("Please enter your phone number."); isValid = false; } else if (!isValidNumber(number.value)) { alert("Please enter a 10-digit phone number."); isValid = false; } return isValid; }
Initializes a boolean variable isValid to true. This variable will be used to keep track of whether the form is valid or not.
Gets references to the name, email, and number input fields using document.getElementById().
Checks each input field for validity. If an input is invalid, it sets isValid to false and displays an error message using alert().
Returns the value of isValid, which will be true if all input fields are valid and false otherwise. For the name input field, it checks if the value is empty. If it is, it displays an error message and sets isValid to false.
For the email input field, it checks if the value is empty. If it is, it displays an error message and sets isValid to false. If it's not empty, it calls the isValidEmail() function to check if the email address is in the correct format. If it's not, it displays an error message and sets isValid to false.
For the number input field, it checks if the value is empty. If it is, it displays an error message and sets isValid to false. If it's not empty, it calls the isValidNumber() function to check if the phone number is in the correct format (10 digits). If it's not, it displays an error message and sets isValid to false.
Finally, the function returns the value of isValid, which will be true if all input fields are valid and false otherwise. hope that was helpful keep up the great work 🔥
Marked as helpful0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord