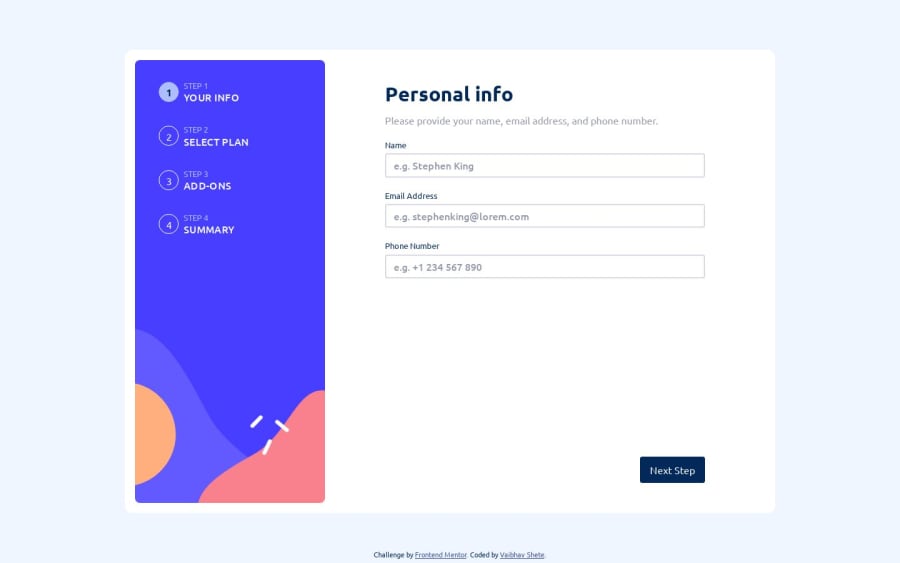
Design comparison
Solution retrospective
I haven't tried to match the design pixel-by-pixel, but instead have tried to understand the look and feel and replicate it.
Pixel-perfect-wise it might be wrong, but what do you think about the feel?
Another question I have is about form validation. How to you go about it in React? Do you too keep all info in a state variable and check it? Is there some frequently used library that could make common validations easy?
Community feedback
- @khaya05Posted over 1 year ago
Hey Vaibhav
Great job on this project. One common way of validating forms in react is to use a library called "react-hook-form" and "yup" if you use javascript, or "ZOD" if you use typescript.
Here is an example of using Yup and react-hook-form:
Step 1: Install Yup and React Hook Form
npm install yup react-hook-form
Step 2: Import the required dependencies
import React from 'react'; import { useForm } from 'react-hook-form'; import { yupResolver } from '@hookform/resolvers/yup'; import * as yup from 'yup';
Step 3: Define your form schema using Yup For example, if you have a form with a name field and an email field, you can define the schema like this:
const schema = yup.object().shape({ name: yup.string().required('Name is required').min(2, 'Name should be at least 2 characters'), email: yup.string().required('Email is required').email('Invalid email address'), });
Step 4: Use React Hook Form with Yup resolver Inside your React component, initialize the React Hook Form and specify the Yup resolver:
const MyForm = () => { const { register, handleSubmit, formState: { errors } } = useForm({ resolver: yupResolver(schema), }); const onSubmit = (data) => { // Handle form submission console.log(data); }; return ( <form onSubmit={handleSubmit(onSubmit)}> <div> <label>Name</label> <input type="text" {...register('name')} /> {errors.name && <p>{errors.name.message}</p>} </div> <div> <label>Email</label> <input type="text" {...register('email')} /> {errors.email && <p>{errors.email.message}</p>} </div> <button type="submit">Submit</button> </form> ); };
In the code above, the
register
function from React Hook Form is used to register the form fields. ThehandleSubmit
function is used to handle form submission. Theerrors
object contains the validation errors from Yup, and it's used to display error messages for the corresponding fields.Step 5: Render the form Finally, render the
<MyForm />
component wherever you want the form to appear in your application.Also, check out the documentation to learn more:
Yup:
React Hook Form:
Hope this helps!👍
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord