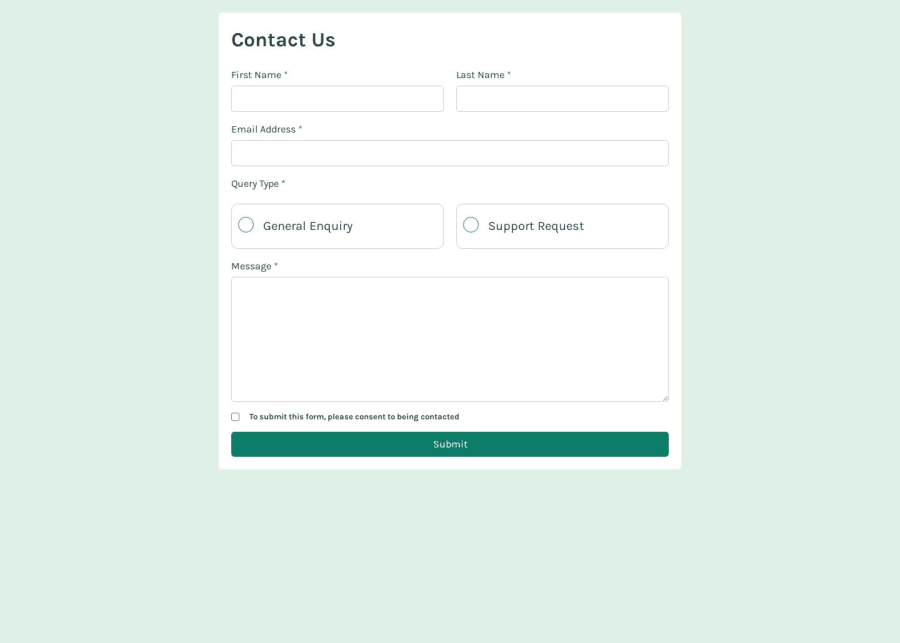
[MOBILE FIRST / SASS] Accessible Responsive Contact Form
Design comparison
Solution retrospective
my code or this app validate and check if all form fields are filled out correctly before submitting. It ensures the first name, last name, message, and email are valid, that a radio button is selected, and that the consent checkbox is checked. If there are errors, it highlights them and shows messages. When everything is correct, it displays a success message and reloads the page after 2.5 seconds. In a real-world scenario, instead of reloading the page, you'd typically handle the form submission with a backend system to process and store the data.
in case you need that code for reference or to use to it to validate your own form feel free to use it
What challenges did you encounter, and how did you overcome them?Validation Logic but with some research i think i did alright Accessibility trying my best to make my app accessible to everyone
What specific areas of your project would you like help with?if you can read my code or just the script file correct my mistakes ill highly appreciate it the most help needed is how I can improve my logic and my code thank you
Community feedback
- @krushnasinnarkarPosted 4 months ago
Hi @AReactDeveloper,
Congratulations on successfully completing the challenge!
Your current JavaScript code for form validation is functional, but there are some improvements you could make for better readability, maintainability, and efficiency. Here are a few suggestions:
Key Improvements:
- Separate Concerns: Create separate functions for different types of validations.
- Reduce Repetition: Use a generic function for showing error messages.
- Consistent Naming: Maintain consistency in variable naming for clarity.
- Use
forEach
for Iteration: If validating multiple elements, consider usingforEach
. - Improve
isValid
Handling: EnsureisValid
is initialized totrue
and only set tofalse
inside each validation function if any validation fails.
Here's a revised version of your code:
// Variables const form = document.getElementById('myForm'); const firstName = document.getElementById('firstName'); const lastName = document.getElementById('lastName'); const email = document.getElementById('email'); const general = document.getElementById('general'); const support = document.getElementById('support'); const message = document.getElementById('message'); const consent = document.getElementById('consent'); const submitMsg = document.querySelector('.succesMsg'); const errorColor = 'hsl(0, 66%, 54%)'; let isValid = true; const resetValidation = () => { isValid = true; document.querySelectorAll('.error').forEach(error => error.style.display = 'none'); document.querySelectorAll('input, textarea').forEach(input => { input.style.borderColor = ''; input.style.outlineColor = ''; }); }; const showError = (input, message) => { input.style.borderColor = errorColor; input.style.outlineColor = errorColor; const errorElement = input.nextElementSibling; errorElement.textContent = message; errorElement.style.display = 'block'; errorElement.style.color = errorColor; isValid = false; }; const validateInput = (input) => { if (input.value.trim() === '') { showError(input, '*Input field required'); } }; const validateEmail = (input) => { const emailPattern = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/; if (!emailPattern.test(input.value.trim())) { showError(input, '*Invalid email address'); } }; const validateRadio = () => { if (!general.checked && !support.checked) { const queryError = document.getElementById('queryError'); queryError.style.display = 'block'; queryError.style.color = errorColor; isValid = false; } }; const validateConsent = (input) => { if (!input.checked) { const consentError = input.parentElement.nextElementSibling; consentError.style.display = 'block'; consentError.style.color = errorColor; isValid = false; } }; form.addEventListener('submit', (e) => { e.preventDefault(); resetValidation(); validateInput(firstName); validateInput(lastName); validateInput(message); validateEmail(email); validateRadio(); validateConsent(consent); if (isValid) { submitMsg.style.display = 'block'; setTimeout(() => { location.reload(); }, 2500); } });
Key Changes:
- Modular Functions: Validation logic is separated into individual functions.
- Centralized Error Handling: Error handling is managed by the
showError
function. - Validation Reset: Added a
resetValidation
function to clear previous errors. - Consistent Error Display:
isValid
is used consistently to track validation status.
These adjustments should help make your code cleaner and more manageable.
I hope you find this helpful.
Feel free to reach out if you have more questions or need further assistance.
Happy coding!
1@AReactDeveloperPosted 4 months agoThank you for your comment, @Krushnasinnarkar. I view functions as reusable blocks of code, and in my function to validate input, I reused the same line of code by placing it in a function for reuse throughout the code, which I found beneficial. I considered doing the same for validating email, radio buttons, and consent checkboxes, but it felt excessive to remove the code, rewrite it into a function, and then call it in the same place without reuse. While this approach may be worthwhile in a larger application, it seemed unnecessary for a small form.
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord