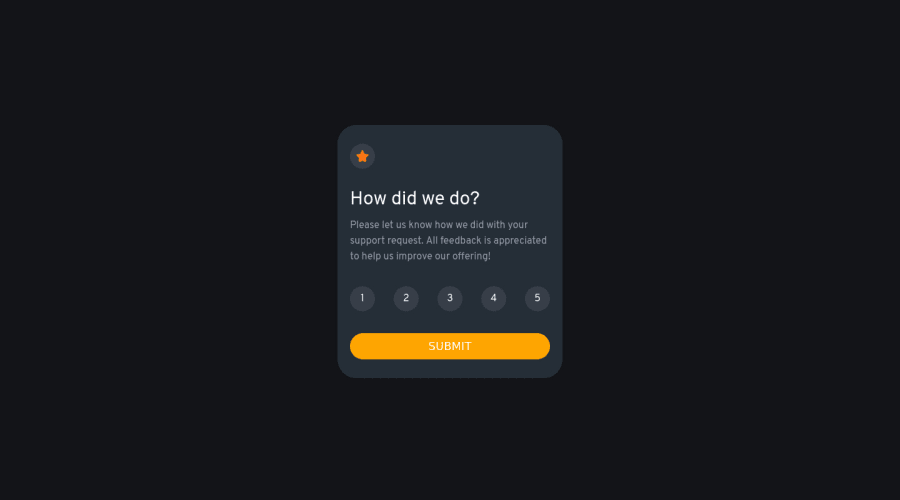
Design comparison
SolutionDesign
Solution retrospective
Dear community members,
This is the second challenge. I have developed the interactive rating component. Please review the code in the repository and provide possible feedback on my code structure and readability.
Thanks & regards,
Khalil
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord