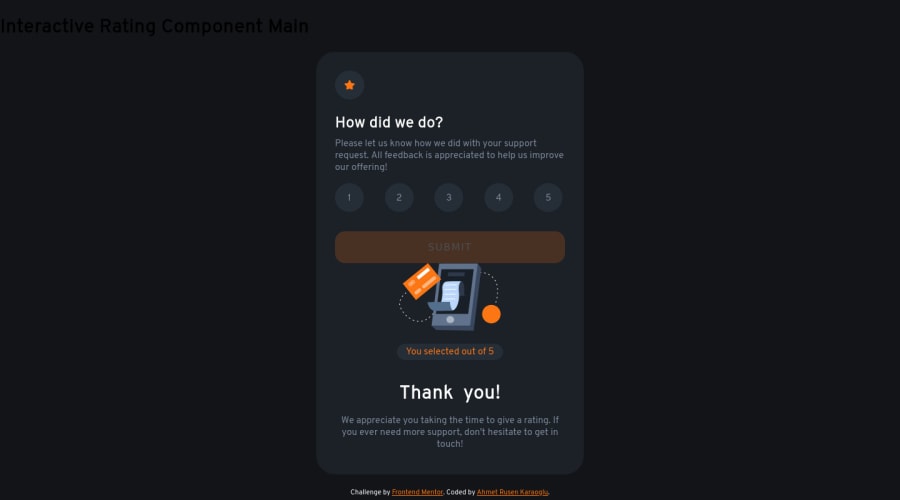
Responsive Interactive Rating Component
Design comparison
Solution retrospective
This challenge was a good learning experience for me overall.
If there is another efficient way, I would like to know if I can write a for loop of some sort to make is less tedious to write JS event listeners to Rating Buttons 1-5.
Any feedback is welcome. I have thick skin so please don't shy away from giving constructive criticism.
Community feedback
- @Osama-ElshimyPosted over 2 years ago
Nice work!
Here are how I did the JS event listeners:
- Select the parent element of the rating buttons like you did my friend:
const ratingList = document.querySelector(".rating-group"");
- Select all the rating buttons together:
// This will choose all the button elements and give you a Node List const ratingBtns = document.querySelectorAll(".rating-btn");
- Select the span element with the
rating-value
class:
const selectedRate = document.querySelector(".rating-value");
- Select the form:
const form = doqument.getElementById('form');
// Select rating handler // 1. Add event listener to common parent element - (ratingList) ratingList.addEventListener("click", function (e) { e.preventDefault(); // 2. Determine what element caused the event - Very important step const clicked = e.target.closest(".rating-btn"); // Gaurd Clause - return if no button is clicked if (!clicked) { return; } // console.log(clicked); // Remove active class from all buttons ratingBtns.forEach(btn => btn.classList.remove("active")); // Add active class to clicked button clicked.classList.add("active"); // Assign rate to clicked button value selectedRate.textContent = ` ${clicked.textContent}`; }); // Submit form form.addEventListener("submit", function (e) { e.preventDefault(); // Make sure a rating is selected if (!selectedRate.textContent) { console.log(`You must Choose a rate!`); return; } console.log("Submitted"); // Hide rating container ratingContainer.classList.add("hidden"); // Display thank container thankContainer.classList.remove("hidden"); });
The rating part is possible because of something called
event propagation
. You can read more about that here:[Event Propagation] (https://www.tutorialrepublic.com/javascript-tutorial/javascript-event-propagation.php)
Overall that was a great job on the UI, you just need to refactor the JS code.
If you find this helpful, click on the Mark as helpful button.
Happy Coding
Marked as helpful0 - P@ARKaraogluPosted over 2 years ago
Hi everyone,
Please excuse the screenshot above. For some reason my hidden contents are also being made visible in the screenshot.
- Ahmet
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord