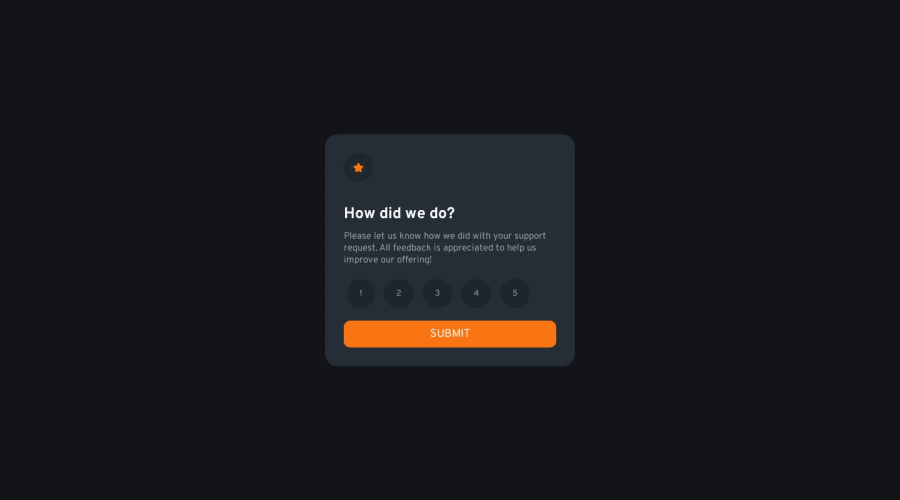
interactive rating component built using HTML, pure CSS and vanilla JS
Design comparison
Solution retrospective
Hello everyone,
I just completed this interactive component. I used pure CSS and Vanilla JS. I used a lot repeated codes in my JS so as to achieve the functionalities. This is because I am just learning the basics of JavaScript. However, I know there are better and concise way of achieving same effect.
If you come across this solution, please spare some minutes to go through my codes and drop comments on them. Especially, what I can learn to write better codes.
Thank you.
Community feedback
- @3eze3Posted over 1 year ago
Hi Soph-iee very good project for this challenge, I see that you had complications in your JavaScript. Before I check and help you to remove the duplication in your code.
I want to see your HTML structure:
- There is not
<h1></h1>
in the html is understandable as it does not have an impact with the design , but semantically it is , and in terms of accessibility and SEO.(While it is a practice , it is good to keep this in mind).
-The use of semantic tags such as a
<main></main>
for card/component, is better structurally, as they have more semantic meaning than a .<div>.- The image :
<img src="images/illustration-thank-you.svg" alt="thank you" />
it is not necessary to use the atl, you could use an aria-hidden:true, as they are just decorative.
The section with which the user interacts:
<div class="rating-word"> Please let us know how we did with your support request. All feedback is appreciated to help us improve our offering! </div> <button class="ratings one unclicked">1</button> <button class="ratings two unclicked">2</button> <button class="ratings three unclicked">3</button> <button class="ratings four unclicked">4</button> <button class="ratings five unclicked">5</button> <button type="button" class="submit-btn">Submit</button> </div>
The use of buttons or inputs, may vary according to the project, but for this one it is more convenient to use inputs instead of buttons.
Buttons can be beneficial when you need them:
-
You want to allow users to select multiple options from the 5 available, rather than a single option.
-
Each option has an immediate action associated with it, and you don't want to wait for the user to click a submit button after each selection.
But that's not what we're looking for with this project, so it would be better to use radio type inputs. why?:
- The user is mutually exclusive, they can only select one of the options and not several at the same time.
- You can evaluate the user inputs with only one function and not use 5 functions to evaluate each user click.
So looking at these points the structure could look like this :
<form action="#" class="card__form"> <fieldset class="card__options"> <legend class="card__title hidden">rate us from 1-5:</legend> <input class="card__check" type="radio" name="options" id="option-1" /> <label class="card__option" for="option-1"> 1 </label> <input class="card__check" type="radio" name="options" id="option-2" /> <label class="card__option" for="option-2"> 2 </label> <input class="card__check" type="radio" name="options" id="option-3" /> <label class="card__option" for="option-3"> 3 </label> <input class="card__check" type="radio" name="options" id="option-4" /> <label class="card__option" for="option-4"> 4 </label> <input class="card__check" type="radio" name="options" id="option-5" /> <label class="card__option" for="option-5"> 5 </label> </fieldset> <button class="card__button" type="submit">SUBMIT</button> </form>
Css:
-
It is better to integrate in your styles the use of relative measures (rem, em, %) instead of absolute ones like (px).
-
You could use some methodology to apply styles and improve the way of naming classes and consequently the structure of your Css styles (You could start with the use of BEM)
If you are interested : Bem Methodology
JavaScript: One thing I can notice is that you focused a lot more on the code itself, but if you look closely, simply using the Html structure radically simplifies the javaScript code.
Variable declaration:
const SubmitButton = document.querySelector('.submit-btn'); const oneStar = document.querySelector('.one'); const twoStar = document.querySelector('.two'); const threeStar = document.querySelector('.three'); const fourStar = document.querySelector('.four'); const fiveStar= document.querySelector('.five'); const rated= document.querySelector ('.selected-number'); const ratingPage = document.querySelector ('.rating-div'); const thankYouPage = document.querySelector ('.thank-you-page');
You can abstract the logic, for example: What happens when a user clicks on btn 1? and on btn 2...? We take a number and add it to the back card.
-
You can use a
querySelectorAll('options')
to select the inputs since you have the same functionality. -
So if you have the same functionality we should not create a function for each button, just a function for the 5 inputs, that will be activated when we click on the submit button.
Look at this is the simplified code:
const $card = document.querySelector(".main__wrapper"); const $button = document.querySelector(".card__button"); const $rating = document.querySelector(".card__rating"); const $options = document.querySelectorAll(".card__check"); function setRating() { let selectedOption = Array.from($options).find(option => option.checked); let message = `You selected ${selectedOption.nextElementSibling.textContent} out of 5`; $rating.textContent = message; } function hangle(event) { event.preventDefault(); $card.classList.add("main__back"); } $button.addEventListener("click", hangle); $options.forEach(option => option.addEventListener("change", setRating));
Obviously this is only one of many solutions that exist, you can modify or use any part of the code that interests you, no answer is absolute and no code is perfect.
I hope this comment is useful not only for this project but for the following ones, although it will take you more time to take all these recommendations, it is better (It is my opinion and it can change).
Greetings and enjoy the process. 🥞
0@Soph-ieePosted over 1 year ago@3eze3 Thank you so much for your time, comments and your recommendations.
They're of great help.
0 - There is not
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord