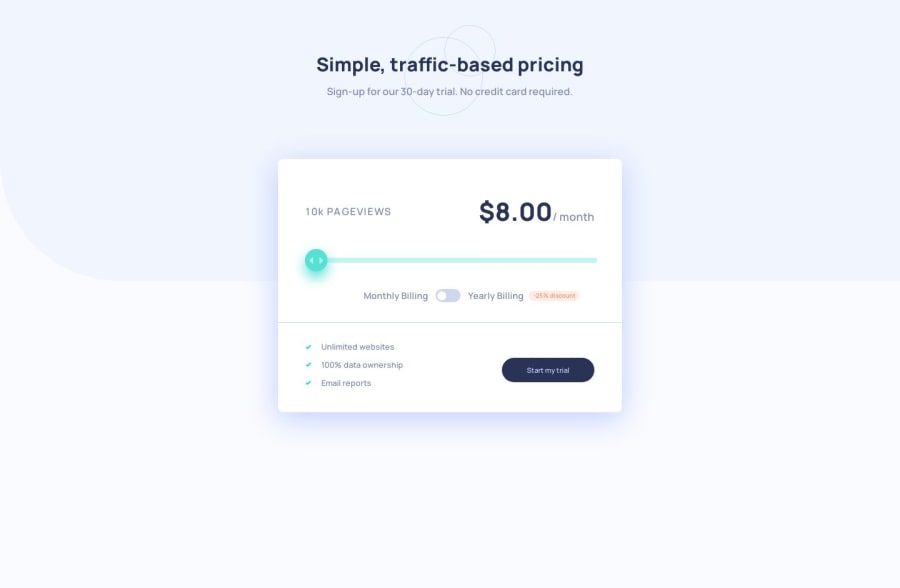
Design comparison
SolutionDesign
Solution retrospective
I had a little tough time with this project since I am new to React, but it was a nice project to tackle. What could I have done better? 🤔
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord