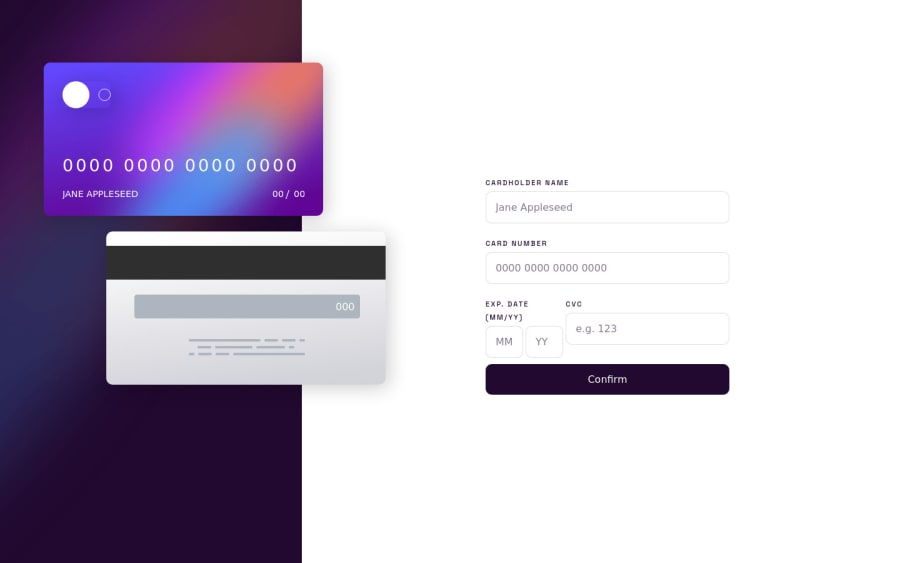
Design comparison
Solution retrospective
In this project I was able to practice concepts of regular expressions and apply Sass. I feel like I need to improve these techniques and make my code cleaner.
What tips would you give me to improve validation, to separate the numbers into groups of 4 and to make the Sass code cleaner?
const validation = { name: /\d/g, number: /\D/g, }
Community feedback
- @jessegoodPosted about 2 years ago
Nice solution!
Here are some tips to improve your code.
-
To improve validation: It seems you are performing validation on the
keyup
event. However, the form can still be submitted even if the form is not filled out. I would suggest listening to thesubmit
event, perform validation and only allow submitting the form when there are no errors. -
I think learning the built in JavaScript Validation API would be good. For example, you can set the
required
attribute to input forms and then just checkinput.validity.valueMissing
to see if the field is blank. -
Putting numbers into groups of 4: Here is the function I use. You could listen to the
input
event on your input field and then format the input using the function below.
function formatCreditCardNumber(num) { num = num.split(" ").join(""); let arr = num.split(""); let formattedNum = []; if (arr.length < 4) return num; for (let i = 0; i < arr.length; i++) { if (i !== 0 && i % 4 === 0) { formattedNum.push(" "); } formattedNum.push(arr[i]); } return formattedNum.join(""); }
For improving your SASS, I highly recommend looking into nesting to make your CSS more compact and readable: Here is a tiny example using your code. However,
.container { .aside { width: 100%; height: 40vh; background-repeat: no-repeat; background-size: cover; .front-card { position: absolute; top: 70%; left: 10%; z-index: 1; } } }
The above compiles to:
.container .aside { width: 100%; height: 40vh; background-repeat: no-repeat; background-size: cover; } .container .aside .front-card { position: absolute; top: 70%; left: 10%; z-index: 1; }
Also, to space our your numbers, use
font-variant-numeric: tabular-nums;
!0 -
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord