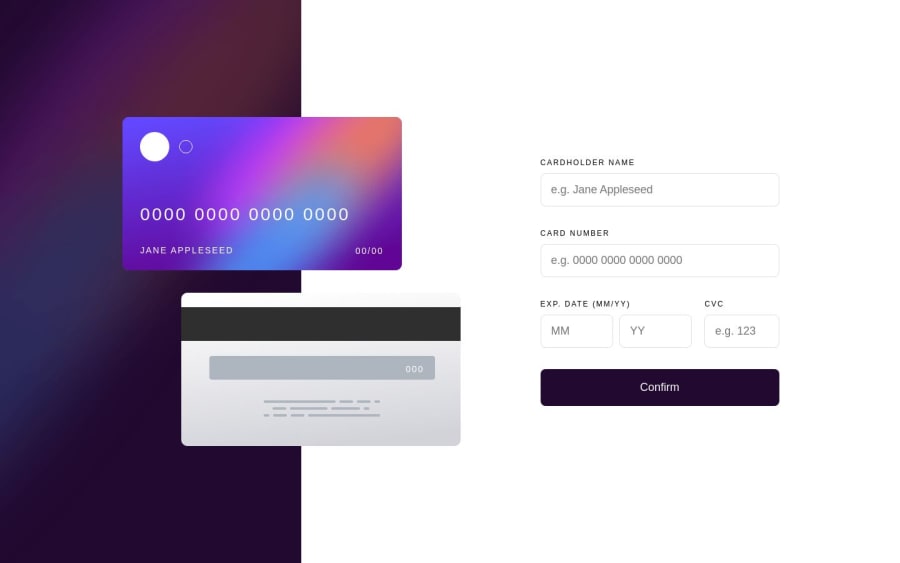
Interactive Card Details Form Main ( HTML, CSS, JS, SASS, RESPONSIVE)
Design comparison
Solution retrospective
- Variable Declarations: javascript Copy code // DOM Elements const confirm = document.getElementById("confirm"); const iName = document.getElementById("iName"); const iNumber = document.getElementById("iNumber"); // ... (other input and output elements)
// Modal Elements const continueButton = document.getElementById("continue"); const btnCloseModal = document.querySelector(".close-modal"); const modal = document.querySelector('.modal'); const overlay = document.querySelector('.overlay');
// Form Error Elements const borderStyle = document.querySelectorAll(".form__item--input") You declare variables to store references to various DOM elements, including form inputs, outputs, modal elements, and error elements. The borderStyle variable holds a collection of form input elements for styling purposes.
-
Modal Functions: javascript Copy code // Functions to open and close the modal const openModal = function () { /* ... / }; const closeModal = function () { / ... */ }; These functions control the visibility of a modal dialog. openModal removes the 'hidden' class from modal elements, while closeModal adds the 'hidden' class to hide the modal. The modal can also be closed by clicking outside it or pressing the 'Escape' key.
-
Event Listeners: javascript Copy code // Event listeners for modal interaction continueButton.addEventListener("click", openModal); btnCloseModal.addEventListener('click', closeModal); overlay.addEventListener('click', closeModal); document.addEventListener('keydown', function (e) { /* ... */ });
// Event listeners for form confirmation continueButton.addEventListener("click", closeModal); confirm.addEventListener("click", addDetail); You set up event listeners for various actions, such as button clicks and key presses, to control the modal's behavior and trigger form validation and submission.
- Form Validation and Submission: javascript Copy code // Function to handle form confirmation function addDetail(event) { /* ... */ }
// Function to validate input fields and display error messages function validateAndSetField(value, errorElement, validationFunction, outputElement, borderElement) { /* ... */ } These functions handle the form submission and input validation. The addDetail function is called when the user confirms the form. It retrieves input values, validates them using the validateAndSetField function, and updates the output elements if the input is valid. The validation function checks for various conditions based on the type of input.
-
Input Formatting: javascript Copy code // Format card number input with spaces iNumber.addEventListener("input", function (e) { /* ... */ }); This event listener formats the card number input by adding spaces after every four characters, improving the visual presentation.
-
Helper Functions: javascript Copy code // Helper functions for error messages function showErrorMessage(element, message) { /* ... / } function hideErrorMessage(element) { / ... */ } These functions handle the display and hiding of error messages for better user feedback during form validation.
-
Validation Functions: javascript Copy code // Validation functions function isValidName(name) { /* ... / } function isValidNumber(number) { / ... / } function isValidExpiryYear(year) { / ... */ } These functions define regular expressions to validate the format of the name, card number, and expiry year.
-
Reset Functions: javascript Copy code // Reset functions function resetInputs() { /* ... / } function resetInputField() { / ... / } function resetBorderStyle() { / ... */ } These functions reset the displayed and input values, as well as the border style of form inputs after form submission or when needed.
-
Styling Functions: javascript Copy code function validBorder(element) { /* ... / } function inValidBorder(element) { / ... */ } These functions handle setting the border style of form inputs for visual feedback during validation, indicating whether the input is valid or invalid.
In summary, your code follows a modular structure, separating concerns into functions for better maintainability and readability. It handles user interactions, validates form inputs, and provides clear feedback to the user. The use of comments, meaningful function and variable names, and a well-structured layout contribute to the code's overall readability and understandability.
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord