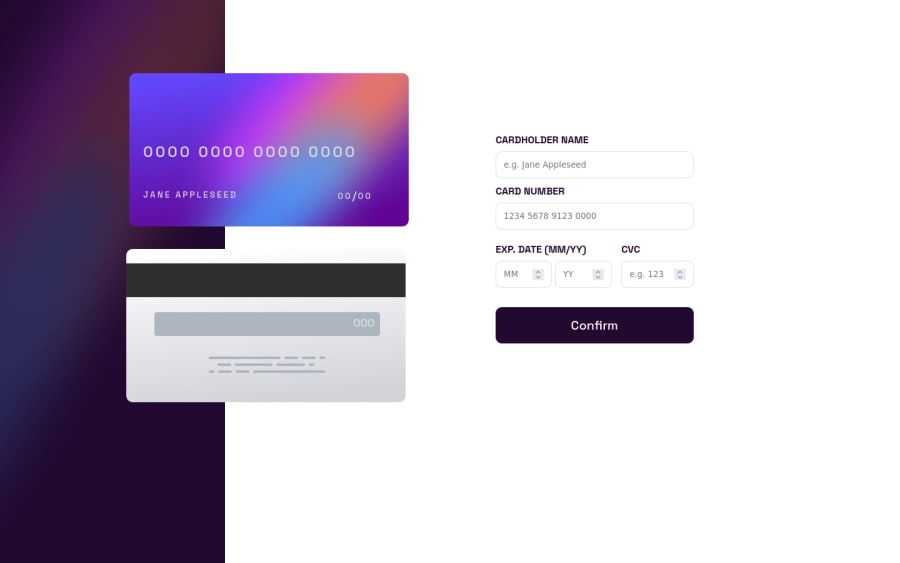
Interactive card details form | HTML, CSS, CSS animation, JavaScript
Design comparison
Solution retrospective
I struggled quite a bit with this project, so feedback on any of the following is welcome:
- Layout
- Responsiveness
- Error message functionality
- Form validation
Community feedback
- @kowsirahmedPosted over 1 year ago
@savvystrider Well done. I will recommend followings:
Logical errors in form validation.
- No errors for name and number are submitted empty.
- No error if number field is invalid.
- When space apears after entering each 4 digit in cardNumber, there is a late.
Validation problems solution.
- HTML: Add an span element after each input with error class
<span class="error-container"></span>
- CSS:
.error { color: var(--input-errors); font-size: 12px; display: none; }
- JS:
- select those errorContainers
const errorsContainers = form.querySelectorAll("span.error-container")
- change cardNumber event from 'keyup' to 'input'
cardNumber.addEventListener("input", function(e) {...}
- modify the submit event handling function
- select those errorContainers
e.preventDefault(); let error = false; // global flag to keep track of any input error so that we can prevent final submission inputs.forEach((input, index) => { // for each input fields check for empty error if (input.value === "") { // display error and set global flag to true errorsContainer[index].textContent = "Can't be blank"; errorsContainer[index].style.display = "block"; error = true; } else { // remove previusly set error. errorsContainer[index].textContent = ""; errorsContainer[index].style.display = "none"; } }) if (cardNumber.value.search(/[^\d\s]/) !== -1) { // check cardNumber has any format error and display erros errorsContainer[1].innerHTML = "invalid format"; errorsContainer[1].style.display = "block"; error = true; } else { // remove previusly set error. errorsContainer[1].textContent = ""; errorsContainer[1].style.display = "none"; } if (error) return; // return if error is found in any input // if no errors show success page form.style.display = "none"; document.getElementById("success-container").style.display = "block";
Layout problem solution
You have to position the card-front and card-back images absolutely inrelation to the card-container. This is a great article for layout. Understanding Layout
In short change the following in css:
.card-container { position: relative; } .front { position: absolute; /* remove self from the regular element flow. position according to the parent which was positioned (in this case .card-container) otherwise position according to body */ top: 50%; /* the distance between .card-container top and .front top is 5% of the .card-container width */ left: 5%; /* the distance between .card-container left and .front top is 5% of the .card-container width */ z-index: 1; } .back { position: absolute; top: 5%; /* the distance between .card-container top and .back top is 5% of the .card-container width */ right: 5%; /* the distance between .card-container right and .back top is 5% of the .card-container width */ }
Similarly card front and back content should be positioned.Also fix those html warning and errors given by frontendmentor.
Marked as helpful1@savvystriderPosted over 1 year ago@kowsirahmed Thank you for taking the time to look through my code! Your suggestions helped me improve it quite a bit
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord