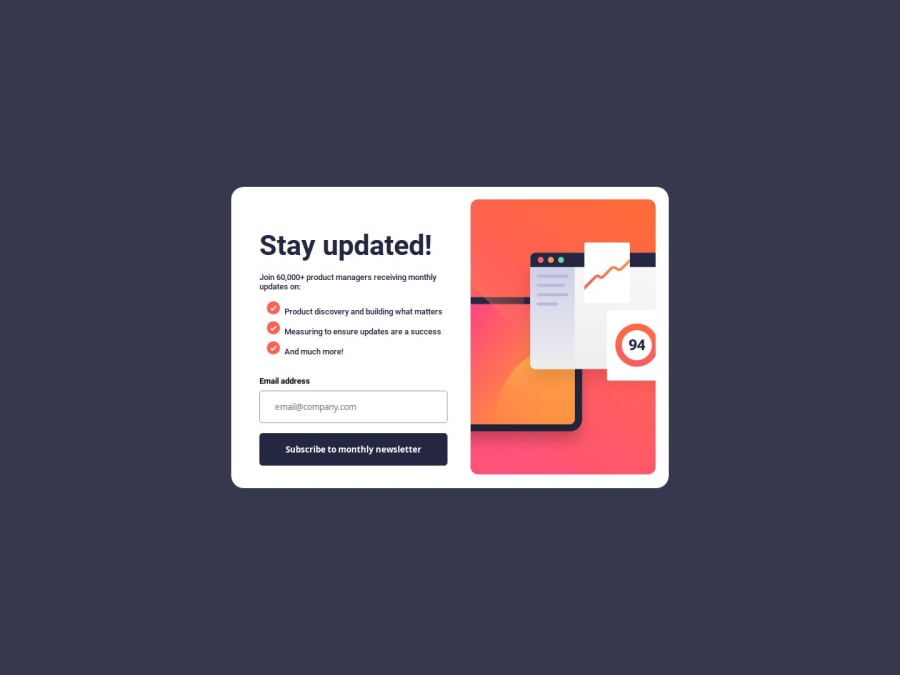
Design comparison
Solution retrospective
can anyone help me with the css validation? like the :invalid and the :invalid state I try using this but the default of this is sucks like it automatically invalid even though user still didn't do anything. I try user-invalid and user-valid and it work fine and it work as expected. Is it good using that tho?
Community feedback
- @NikitaVologdinPosted about 2 months ago
Hi @Hoaxilog! I suggest not to use browser validation. Because the task in that challenge is to use JS validation. Here you can find out about client-side validation: https://developer.mozilla.org/en-US/docs/Learn/Forms/Form_validation#different_types_of_client-side_validation
For that add novalidate attribute on form tag in html. You can read about attribute here: https://developer.mozilla.org/en-US/docs/Web/HTML/Element/form#novalidate
After all that:
- We need to get submitted information:
const handleSubmit = (e) => { e.preventDefault(e); const formData = new FormData(e.target); const data = Object.fromEntries(formData); console.log(data); };
Now we have submitted data from all inputs (we have only one, but you can use the same approach if you have more).
- We need to check the validity of email input in JS:
function validateEmail(email) { const regExp = /^[\w-\.]+@([\w-]+\.)+[\w-]{2,4}$/g; // this is regular expression return regExp.test(email); // here we are testing submitted email. // result is true or false }
2.1 We can store result of the function in variable:
const isEmailValid = validateEmail(data.email)
3. Now we can do something according isEmailValid variableIf(isEmailValid){ //If valid show dialog } if(!isEmailValid) { //If not valid show error }
Final code might look like this:
function validateEmail(email) { const regExp = /^[\w-\.]+@([\w-]+\.)+[\w-]{2,4}$/g; // this is regular expression return regExp.test(email); // here we are testing submitted email. // result is true or false } emailForm.addEventListener("submit", (e) => { e.preventDefault() const formData = new FormData(e.target); const data = Object.fromEntries(formData); const isEmailValidated = validateEmail(data.email) if(isEmailValidated) { show dialog } if(!isEmailValidated) { show error } })
No need to use action="#success-message" method="get" on form. Those are for php script.
Marked as helpful1@HoaxilogPosted about 2 months ago@NikitaVologdin thankyou for detail explanation. I will re-code my js code
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord