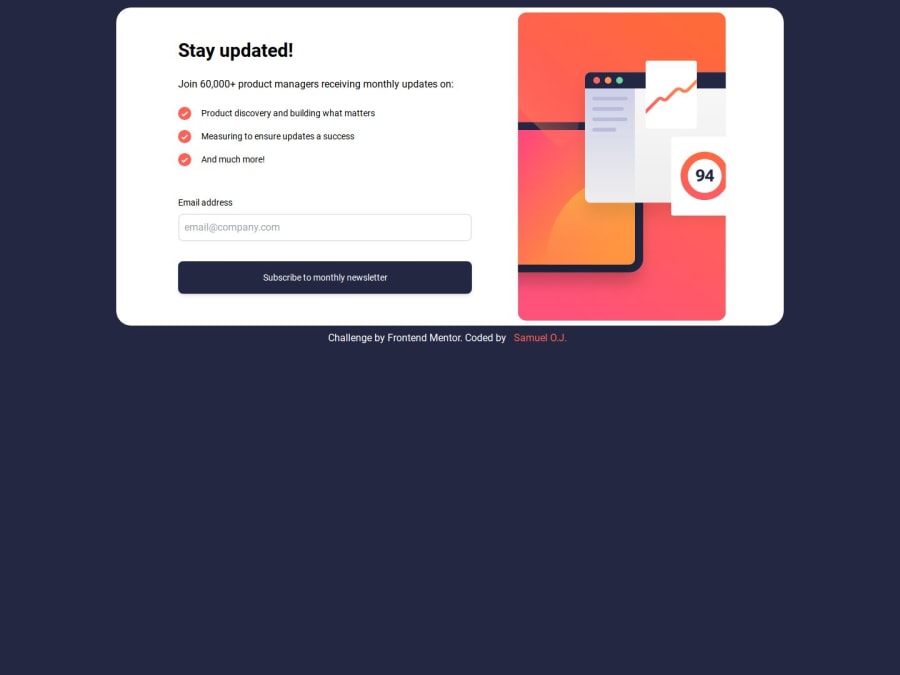
Design comparison
Solution retrospective
- prevent default Dom submission and I couldn't implement that aspect as the firm can still be submitted without any input.
Please log in to post a comment
Log in with GitHubCommunity feedback
- @RazaAbbas62
It looks like you have a form submission event listener in your JavaScript code that should handle the form submission. However, the issue may be related to the
validateEmail
function, which is currently commented out. Also, the event listener for the form submission is preventing the default behavior, but you need to explicitly check if the email is valid before proceeding with the submission.Here's an updated version of your code with a simple email validation check using a regular expression:
// ... // function to validate email using a regular expression function validateEmail(email) { const regex = /^\S+@\S+\.\S+$/; return regex.test(email); } // form event listener form.addEventListener('submit', (e) => { // prevent default form submission e.preventDefault(); // get the email value from the input field const emailValue = mailInput.value.trim(); // check if the email is valid if (validateEmail(emailValue)) { // update the user email on the success page updatedUserEmail(emailValue); // clear the input field mailInput.value = ""; // toggle between the success page and home page switchPages(); } else { // if the email is not valid, display an error message error.textContent = 'Enter a valid email address'; } }); // ...
This code uses a simple regular expression to check if the entered email is in a valid format. If the email is valid, it proceeds with the form submission logic; otherwise, it displays an error message. Adjust the regular expression based on your specific email validation requirements.
Enjoy coding :)
Marked as helpful
Join our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord