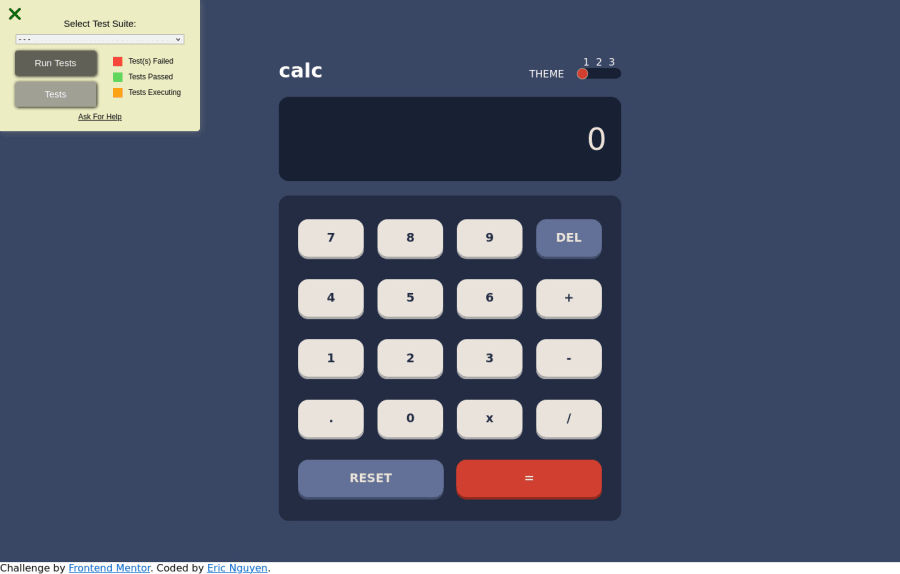
Calculator application using create-react-app with hook
Design comparison
Solution retrospective
This is my calculator application using create-react-app You guys can check my result at domain: https://calculator-react-app-psi.vercel.app/ Feel free to comment if you want to improve anything in this product. Thanks for visiting my repo!
Community feedback
- @kem522Posted over 3 years ago
Heya! Good work! Very nice use of the useState hook.
I have a couple of pieces of advice mostly surrounding the buttons on the calculator.
You've used a
div
for those buttons but making thembuttons
is semantically preferable as it is more descriptive of what they are and it comes with accessibility benefits, the most important of which is that button elements are keyboard focusable. A user who can only use keyboard can use thetab
key to move through interactive elements on the page, divs are not focusable by default but buttons are.Another benefit of using the button element is that you can leverage the
value
attribute in the click events and usinge.target.value
to pass in the value to thehandleNumbers
function, would look something like this:// the button would now just take handleNumbers as the onClick callback // I also added a value attribute equal to the value i'd expect this button to have on the calculator <button onClick={handleNumbers} className={`padButton maths ${theme==='2'?"text-color-2 btn-color-2":theme==='3'?"text-color-3 btn-color-3":""}`} id="seven" value="7">7</button> // in the handle numbers function you would use e.target.value const handleNumbers = (e) => { const number = e.target.value; if(currentVal.length >= 21) { alert('Digit Limit Met!'); } else { if(expression === '0') { deleteBack(); } display(number); } setCurrentVal(prev => prev + number); }
In the example above, the only thing that changes between the button numbers is the text in the button and the value attribute, so you could use an array of numbers and a map to create the buttons instead of having to do them one by one:
{[0, 1, 2, 3, 4, 5, 6, 7, 8, 9].map((num) => { return ( <button onClick={handleNumbers} className={`padButton maths ${ theme === "2" ? "text-color-2 btn-color-2" : theme === "3" ? "text-color-3 btn-color-3" : "" }`} key={`button-${num}`} value={num} > {num} </button> ); })}
I was playing around with a very simple version of your app (it just logs the numbers in the console) in this codesandbox to make sure I was giving you the correct advice so feel free to take a look at it as it might be more helpful than trying to read the code here: https://codesandbox.io/s/vibrant-hill-bwbkv?file=/src/App.js
Happy coding!
Marked as helpful2@EricNguyen1206Posted over 3 years ago@kem522 Your comments are very helpful to me. Thanks very much!
0@alex-kim-devPosted over 3 years ago@kem522 wow! that's an extensive feedback, well done Katie!
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord