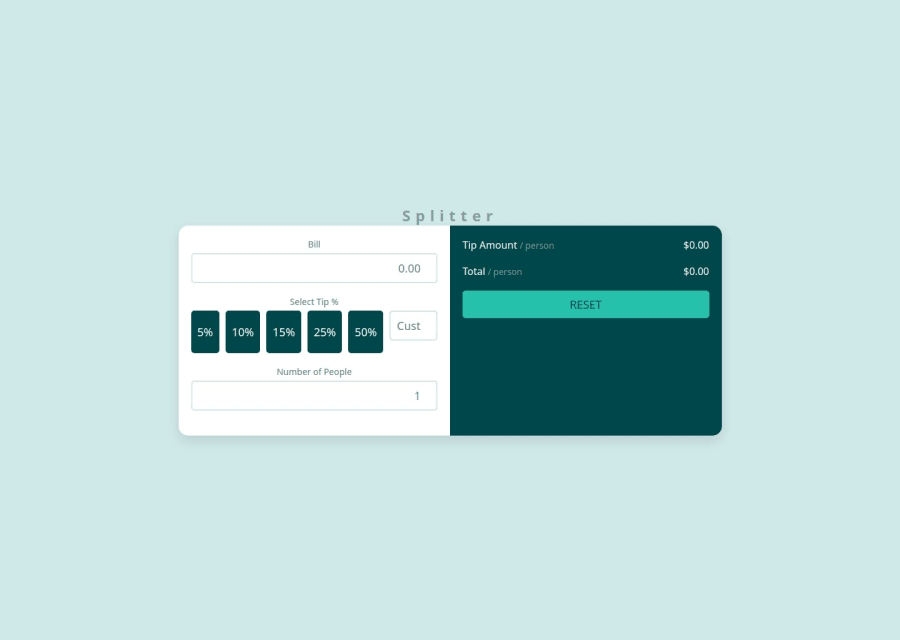
Design comparison
Community feedback
- @RohloffmeisterPosted 16 days ago
HTML Structure
The HTML is well-organized and uses semantic elements effectively:
- Proper use of
<!DOCTYPE html>
and correct language declaration - Logical structuring with
<div>
elements for containers and sections - Appropriate use of form elements like
<input>
and<button>
- Clear separation between input and output areas
Potential improvements:
- Utilize
<form>
for input fields to enhance semantics - Implement
<fieldset>
and<legend>
to optimize form field grouping
CSS Styling
While the CSS creates a visually appealing layout, there are several areas that need improvement:
- Overreliance on pixel units (
px
) for sizing, which can hinder accessibility and responsiveness - Lack of CSS variables for repeated values, leading to potential inconsistencies and maintenance issues
- Absence of media queries, limiting the application's responsiveness on different screen sizes
- Insufficient use of relative units (
em
,rem
) for better scalability - Some redundant declarations that could be optimized
Specific issues:
h1 { font-size: 24px; letter-spacing: 8px; color: #7f9c9c; }
This heading style lacks flexibility. Consider using
rem
for font-size andem
for letter-spacing..inputs, .outputs { padding: 20px; width: 50%; }
Fixed width percentages can cause layout issues on smaller screens. A more flexible approach is needed.
input[type="number"] { /* ... */ font-size: 18px; /* ... */ }
Again, using
px
for font-size limits scalability.rem
would be more appropriate here.Areas for significant improvement:
- Implement a CSS reset or normalize.css to ensure cross-browser consistency
- Use CSS Grid in combination with Flexbox for more complex layouts
- Implement a mobile-first approach with appropriate breakpoints
- Create a modular CSS structure using methodologies like BEM or SMACSS
JavaScript Functionality
The JavaScript code covers the basic requirements and is functional:
- Clear function structuring
- Effective event handling for user inputs
- Implementation of calculation logic
Opportunities for improvement:
- Use constants for unchanging values
- Implement input validation for more robust error handling
- Consider refactoring to improve code reusability
Overall Assessment
While this code represents a functional implementation of a tip calculator, it has room for improvement, particularly in the CSS. The HTML structure is solid, and the JavaScript fulfills the basic requirements. However, the CSS lacks modern best practices and responsiveness.
For future enhancements, consider:
- Implementing accessibility features (e.g., ARIA attributes for better screen reader support)
- Adding localization features for different currencies and languages
- Thoroughly revising the CSS for better responsiveness and maintainability
The developer shows promise in creating functional web applications but should focus on improving CSS skills and adopting modern web development practices.
// Example of a potential refactoring for the calculation function function calculateTip(bill, tipPercent, people) { if (people === 0) return { error: "Number of people can't be zero" }; const tipAmount = (bill * (tipPercent / 100)) / people; const totalAmount = (bill / people) + tipAmount; return { tipAmount: tipAmount.toFixed(2), totalAmount: totalAmount.toFixed(2) }; }
This refactored function could improve code reusability and facilitate easier testing.
0 - Proper use of
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord