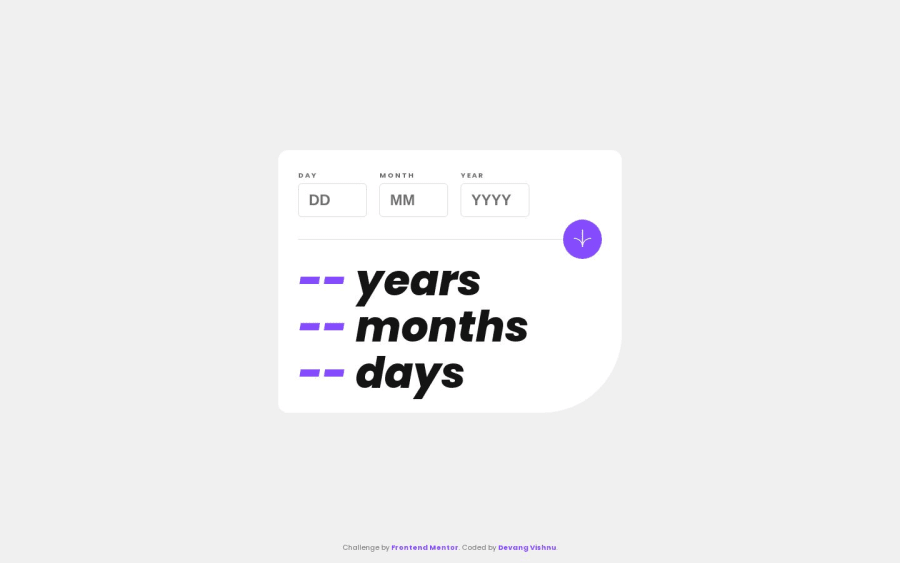
Design comparison
Community feedback
- @ajeetachalPosted about 1 year ago
Devang, your age calculator project is looking fantastic! It's user-friendly and efficient. However, I noticed that the input field currently doesn't prevent users from entering non-numeric values, and there are no error messages displayed in such cases. To enhance the user experience and ensure data integrity, here's a suggestion on how we can improve this aspect:
// Issue: // The input field should only allow numeric values for days, months, and years, and it should also restrict the user from entering invalid data. For instance, days should be limited to 31, months to 12, and years should not be greater than the current year.
// Suggested Solution: // You can achieve this by adding input type and max attributes to the HTML input fields and implementing JavaScript validation. Here's an example code snippet to get you started:
/* HTML: <input type="number" id="day" name="day" min="1" max="31" placeholder="Day"> <input type="number" id="month" name="month" min="1" max="12" placeholder="Month"> <input type="number" id="year" name="year" min="1900" max="2023" placeholder="Year">
JavaScript (Validation): const dayInput = document.getElementById('day'); const monthInput = document.getElementById('month'); const yearInput = document.getElementById('year');
// Add an event listener to validate input on blur (when the field loses focus). dayInput.addEventListener('blur', validateDay); monthInput.addEventListener('blur', validateMonth); yearInput.addEventListener('blur', validateYear);
function validateDay() { const day = parseInt(dayInput.value); if (isNaN(day) || day < 1 || day > 31) { // Display an error message or provide feedback to the user. // You can also reset the input field to a valid value or prevent form submission. } }
function validateMonth() { const month = parseInt(monthInput.value); if (isNaN(month) || month < 1 || month > 12) { // Display an error message or provide feedback to the user. } }
function validateYear() { const year = parseInt(yearInput.value); const currentYear = new Date().getFullYear(); if (isNaN(year) || year < 1900 || year > currentYear) { // Display an error message or provide feedback to the user. } } */
// By implementing these changes, we can ensure that users can only input valid numeric values within the specified ranges, making your age calculator even more user-friendly and error-resistant. Keep up the excellent work!
Marked as helpful0@thedevangvishnuPosted about 1 year agoFirstly, thank you so much for this detailed feedback and the alternative solution/approach that you have shared @ajeetachal I did struggle to code the logic for this age calculator and yes, I knew there were some validation error. Thank you so much for taking our the time to explain this to me. Really appreciate this.
1
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord