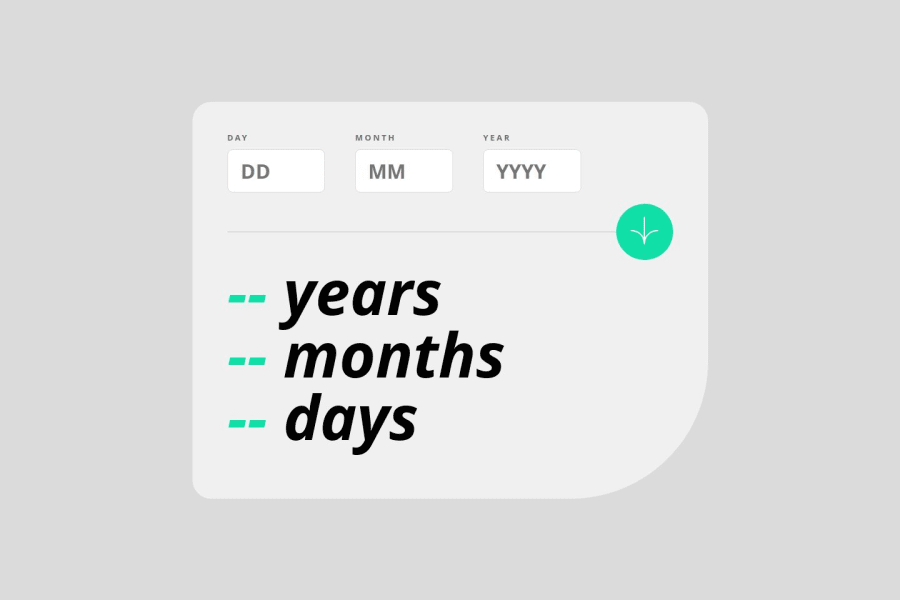
Design comparison
SolutionDesign
Solution retrospective
I struggled a lot with getting the correct time passed since a date because I didn't know what the algorithm should be like, so in the end I chose to count the days between the two dates and divide them by 365.25 to get the years etc. What do you think was the correct approach? Also, I struggled with the 'years', 'months' and 'days' elements in HTML and CSS due to their big font size (they were overflowing). How should I do it in a better way?
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord