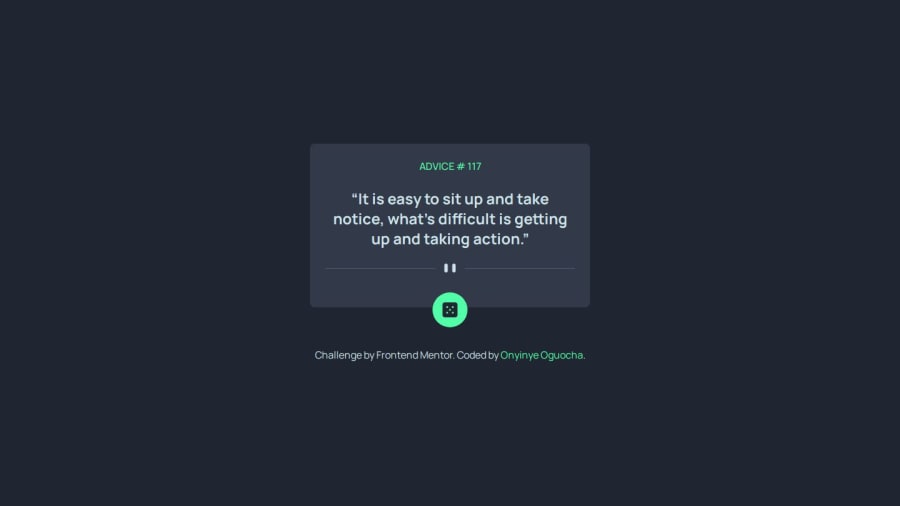
Advice Generator App using React and Tailwind-css
Design comparison
Solution retrospective
I'm proud of Successfully integrating the Advice Slip API while maintaining a meaningful default state. Also, handling API inconsistencies and styling with Tailwind.
Next time, I would plan state management better, clean up unused code earlier, and consider modularizing for better maintainability.
What challenges did you encounter, and how did you overcome them?One of the main challenges I encountered was that the API fetching initially overwrote the default advice on page load. This made it impossible to display the preset advice before fetching new data. I resolved this by adjusting the state handling to ensure the initial advice remained until a new one was fetched.
Another issue was that my project failed to deploy because I had declared useEffect
but never used it. This caused an error, and removing the unused useEffect
fixed the deployment issue.
I would like feedback on optimizing my API fetching logic. Right now, the advice updates when I click the button, but I want to ensure the function is as efficient and clean as possible. Are there better ways to handle this while keeping the code simple?
Community feedback
- @Mahnoor366880Posted about 1 month ago
Hey stephan! you can optimize your API fetching logic:
- Use useEffect for Fetching on Load
Place your API call inside a useEffect hook so it runs when the component mounts.
This prevents unnecessary re-fetching and keeps your component clean.
- Separate Fetch Function
Define a reusable function for fetching advice so it can be used both in useEffect and when clicking a button.
Example:
const fetchAdvice = async () => { try { const response = await fetch('https://api.adviceslip.com/advice'); if (!response.ok) throw new Error('Failed to fetch advice'); const data = await response.json(); return data.slip.advice; } catch (error) { console.error(error); return 'Error fetching advice'; } };
- Handle Loading & Errors
Show a loading message while fetching data.
Display an error message if the API call fails.
- Use React Query for Simplicity (Optional, but recommended for better performance)
A library like React Query makes data fetching cleaner and more efficient.
It handles caching and re-fetching automatically.
Example:
import { useQuery } from 'react-query';
const { data, error, isLoading, refetch } = useQuery('advice', fetchAdvice, { refetchOnWindowFocus: false, });
return (
<div> {isLoading && <p>Loading...</p>} {error && <p>Error: {error.message}</p>} <p>{data}</p> <button onClick={refetch}>Get New Advice</button> </div> );- Keep Button Click Logic Simple
If you’re not using React Query, call your fetchAdvice function inside a button’s onClick event.
Example:
<button onClick={fetchAdvice}>Get New Advice</button>
By following these steps, your code will be more efficient, maintainable, and user-friendly!✨
0
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord