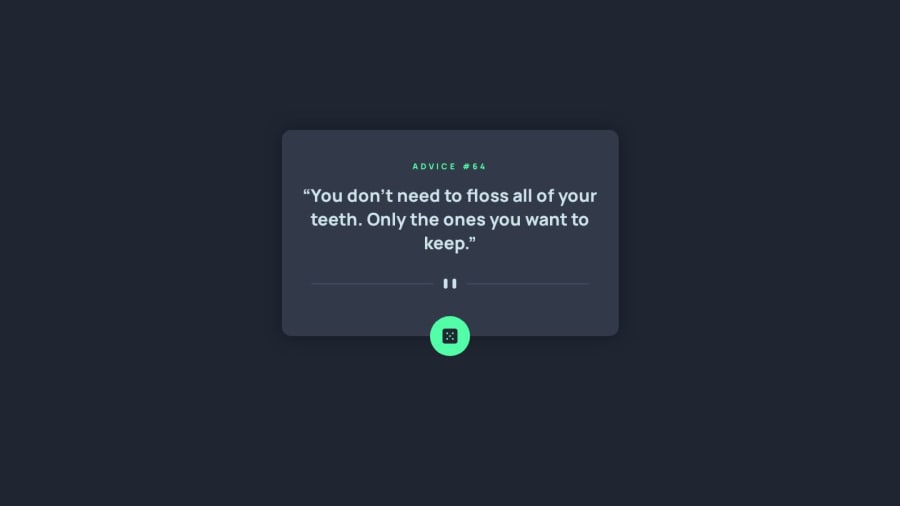
Advice Generator App Creating using React
Design comparison
Solution retrospective
Frontend Mentor - Advice generator app solution
This is a solution to the Advice generator app challenge on Frontend Mentor. Frontend Mentor challenges help me improve your coding skills by building realistic projects.
Table of contents
- Overview
- The challenge
- Screenshot
- Links
- My process
- Built with
- What I learned
- Useful resources
- Author
Overview
The challenge
Users should be able to:
- View the optimal layout for the app depending on their device's screen size
- See hover states for all interactive elements on the page
- Clicking the dice button to check some animation effect on button
- Generate a new piece of advice by clicking the dice icon
Screenshot
Links
- Solution URL: check here🔗
- Live Site URL: check here🔗
My process
Built with
- Semantic HTML5 markup
- CSS custom properties
- Flexbox
- Mobile-first workflow
- React - JS library
- vite
- Vite.js is a development tool that comes with a dev server and is used for modern web applications.
- It offers a faster and smoother workflow in terms of development.
What I learned
-
I used bing chatbot because I have some trouble with React (Because I'm new to React)
-
Debounce - Also learn how to use debounce technic. How it's helps us so much when we're working with very slow API's:
Here is the code:
const handleQuotesGenerator = () => {
getQuotes();
};
const debounce = (callback, delay) => {
let timeOutId;
return function (...args) {
clearTimeout(timeOutId);
timeOutId = setTimeout(() => {
callback(...args);
}, delay);
};
};
const debounceHandleQuotesGenerator = debounce(handleQuotesGenerator, 1000);
return (
<animated.button className="dice" onClick={debounceHandleQuotesGenerator}>
<img src={dice} alt="" />
</animated.button>
);
-
Also learn how learn how to React-Spring. Such a cool library for react users to create beautiful animations without scratching your head.
-
Already learn how to use custom hooks for react components but never really use it for my own project today I also applied custom hooks for my projects.
Here is the code:
import { useEffect, useState } from 'react';
const useQuote = (API_URL) => {
const [quote, setQuote] = useState(null);
const [isLoading, setLoading] = useState(true);
const [isError, setError] = useState(false);
const getQuotes = async () => {
try {
const resp = await fetch(API_URL);
if (!resp.ok) {
setError(true);
setLoading(false);
return;
}
const data = await resp.json();
setQuote(data);
} catch (error) {
setError(true);
console.error(`Error: ${error}`);
}
setLoading(false);
};
useEffect(() => {
getQuotes();
}, []);
return { quote, isLoading, isError, getQuotes };
};
export default useQuote;
Useful resources
Author
- Github - TheRedBandiCoot
- Frontend Mentor - @TheRedBandiCoot
- Twitter - @subho19996
Community feedback
Please log in to post a comment
Log in with GitHubJoin our Discord community
Join thousands of Frontend Mentor community members taking the challenges, sharing resources, helping each other, and chatting about all things front-end!
Join our Discord